A brief update on Angular 2
Last week I watched a very interesting Pluralsight webinar where Joe Eames introduced Angular 2 and I wanted to share what I learned from it with you.
A couple (or four) weeks ago Angular 2 went into developer preview with a beautiful brand new documentation and distributions that you can start playing with today. I hadn’t even looked at it to be honest, busy with other stuff, but I had kept my ear out for news and when pluralsight announced a webinar on the topic I thought it was the perfect time to find out more.
This is what I learned:
Angular 2 is in a very early stage of the developer preview, very unstable and it is constantly changing not only in the form and shape of the API but also in its core concepts and naming.
With Angular 2 you get everything you know and love from Angular 1 with a highly improved performance, a simpler mental model with fewer moving pieces and better access to the DOM.
You can build Angular 2 applications in plain ES5 JavaScript but you also have the option to use transpilers and write your angular 2 apps with TypeScript, ES6/ES7 (traceur, babel…), CoffeeScript, etc.
The whole development story changes from using a standard MVC approach to a more componetized development where you have independent components and each has its template and underlying view model (like in aurelia). The components themselves are just ES6 classes that expose APIs to be bound to templates (no more $scope
variable).
Angular 2 uses ES7 decorators to provide metadata to your components. They work sort of like attributes in C#. If you use ES5 to write your angular apps there’s alternative APIs to define this metadata. This is an example of a component from Joe Eames’ code sample on GitHub that can give you an idea about how the new component model looks like:
// ES 6 module imports
import { Component, View, For, If, EventEmitter } from 'angular2/angular2'
import { Inject, bind } from 'angular2/di'
import { todoItems } from 'services/todoItems'
// component metadata (element and dependencies to inject)
@Component({
selector: 'todo-list',
injectables: [bind('todoItems').toValue(todoItems)],
})
// view metadata (template and used directives)
@View({
templateUrl: 'components/todo-list.html',
directives: [For, If],
})
// an ES6 class
export class TodoList {
constructor(@Inject('todoItems') todoItems) {
this.items = todoItems
}
setCompleted(item, checked) {
item.completed = checked
}
completeAll() {
var that = this
this.items.forEach(function(item) {
that.setCompleted(item, true)
})
}
removeItem(item) {
this.items.splice(this.items.indexOf(item), 1)
}
}
There is some interesting new notation for writing bindings within the html templates (*for
instead of ng-repeat
, *if
instead of ng-if
, binding with [attribute]
and (event)
:
<div style="margin-bottom:10px">
<h1>To Do</h1>
<div style="padding:5px" *for="var item of items">
<input type="checkbox" #chkbox [checked]="item.completed" (click)="setCompleted(item, chkbox.value)">
{{item.text}} <a class="glyphicon glyphicon-remove" (click)="removeItem(item)"></a>
</div>
<button *if="items.length > 1" class="btn btn-xs btn-warning" (click)="completeAll()">Complete All</button>
</div>
The IoC story looks like is more explicit and less convention-over-configuration based although I have no clue how it will look in the final version:
// extract from previous example with IoC fragments
@Component({
injectables: [bind('todoItems').toValue(todoItems) ]
})
// an ES6 class
export class TodoList {
constructor(@Inject('todoItems') todoItems) {
this.items = todoItems;
}
//...
You can start testing the developer preview by downloading the source code at code.angularjs.org (look for the 2.0.x and alphas). In terms of good editor support, there is sublime text that has great plugins for ES6 and ES7, Web Storm, and even Visual Studio Code or Visual Studio if you want to try Angular 2 with TypeScript.
Watch the full webinar with much more interesting information and examplesin youtube:
And here you have the announcement of Angular 2 at ng-conf 2015:
I don’t know why people were so jumpy about breaking changes, this is looking gooood! Hope you enyojed the article! Have a nice day! :)
References
For more information about Angular 2 take a look at:
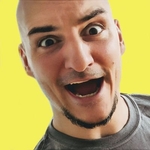
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.