Barbarian Meets Aurelia: First Contact And Building Athena, My Very Own Jarvis
The “barbarian meets” series are a collection of articles that intend to introduce and explain useful libraries, frameworks, tools and technologies in simple and straightforward terms. These new series will focus on the brand new framework from Rob Eisenberg the maker of Durandal and Caliburn frameworks which brings all the goodness of ES6, ES7 and web standards to your toolbelt: Aurelia
This week Rob Eisenberg surprised us all (or me at least xD) when he announced his new web framework Aurelia, a framework that allows you to start using the latest and greatest in JavaScript and web standards to build your apps today.
Aurelia
Aurelia is a bleeding edge web framework that lets you use ES6 and ES7 features today. That means that you can start enjoying all the cool stuff that is coming to JavaScript and to the Web right now this very moment. But let Rob Eisenberg show you much better than I ever could:
Setting Up Your Development Environment
Let’s get started. First, you are going to need to do some installing, you’ll need node, npm and the jspm (the JS package manager for the ES6 module loader). I am going to take a little bit of time with this because even though I use node and npm on a daily basis, it took me a while and some frustration to get aurelia running on my pc and mac.
Windows
If you are using Windows, I will advice you to install node using chocolatey and the PowerShell terminal:
- Get chocolatey, the Windows package manager
- Open your PowerShell console (check out cmder if you haven’t seen it yet) and install node with chocolatey
# cinst is a shortcut for chocolatey install
PS> cinst nodejs.install
If you are not very much into powershell you can use the installer that you can find at nodejs.org.
Node will install npm automatically into your system but it comes with a superold npm version. In order to update npm run this command:
# use npm to update npm xD
PS> npm install -g npm
Verify that you have the latest version by running npm -v
.
PS> npm -v
1.4.2
And that is not the version you want. By default there’s an annoying problem in windows when updating node/npm, you can get all the details here, but in summary node’s npm installation masks the updated version of npm. Just go to your node installation folder, usually Program Files\node
and remove npm
and npm.cmd
. After doing that you should see the right version:
PS> npm -v
2.4.1
Important: For those that already have node and npm in your machine you will need to update it to the very latest version. I couldn’t run jspm
because I was two minor releases behind with node (0.10.34
as opposed to 0.10.36
). So update to the latest version. If you are using chocolatey it will be as easy as chocolatey update nodejs.install
. (Note that you’ll need to remove npm
and npm.cmd
from the node installation folder since these files will be re-created after updating node).
3.Install jspm, the js package manager for ES6 modules:
PS> npm install -g jspm
4.Install gulp, the js task manager that you’ll use for transpiling you ES6 code into vanilla javascript, prepare a distribution of your code, host your app in a server and watch changes in your files among other things:
PS> npm install -g gulp
Mac
If you have a mac:
- Get homebrew, the Mac package manager
- On your terminal (bash, zsh, etc)
$ brew install node
$ npm install -g npm
$ npm install -g gulp
$ npm install -g jspm
Creating an App
Now that you have all the tools that you need, you can either follow the Get Started tutorial at Aurelia.io to boostrap your app or use a yeoman generator to handle everything for you.
The Get Started tutorial involves a lot of downloading files from the web which feels a little bit awkward if you have used yeoman in the past, but it takes you through the process of creating an app step by step which is pretty nice when you’re starting learning a new framework, and I can definitely recommend it just for this very purpose.
I do not know of any official yeoman generator but I have found out this unofficial one that you can use in the GitHubs (Kudos Zewa666!!). You can save a ton of time by using yeoman:
# install yo - the yeoman scaffolding tool - globally
PS> npm install -g yo
# install the aurelia generator globally
PS> npm install -g generator-aurelia
# create your app
PS> mkdir myFirstApp
PS> yo aurelia
# install npm dependencies
PS> npm install
# install jspm dependencies
PS> jspm install -y
# Run your app
PS> gulp watch
# it will probably be hosted in localhost:9000
# check your terminal
Additionally this generator comes with a subgenerator for creating view/viewModel pairs automatically:
PS> yo aurelia:page about
# this will generate an about.html template and the matching
# view model about.js
My advice: follow the tutorial the first time to get your toes wet, and then use the generator when your start building something for real, or in any subsequent times.
The Development Experience
Once you have everything setup you need to start writing some code. You will soon find out that Visual Studio doesn’t provide very good support for writing ES6 style JavaScript (or perhaps there is and I just haven’t found the way, if you know a way please feel free to share).
Fortunately you can always rely on Sublime Text 3 with the ES6 syntax plugin or WebStorm to get a pleasurable dev experience when writing ES6 JavaScript.
Enter Athena
Finally! Now You can start writing something with Aurelia! Yahooooo!
I have started a couple of projects recently, one to dig into yeoman and one to learn more about web standards to get a Microsoft certification so I was pretty out of pet-project ideas to test aurelia with. But then I thought about one old dream of mine and having looked at the [speech synthesis api](Web apps that talk - Introduction to the Speech Synthesis API) recently I decided that it was time to build my very own Jarvis (if you are not familiar with Jarvis you might be with Siri or Cortana). And thus Athena - wait for it - An Artificial Thinking Human-like Noetic Assistant, for lack of a better name XDDD.
The code that you write with Aurelia looks pretty clean. In summary, you write view-viewModel pairs and the framework takes care of composing and binding them together at runtime (it feels like writing knockout components or Durandal). For instance, here we have an example for a basic user form mostly taken from aurelia.io:
// ES6 module and class
export class Welcome {
constructor() {
this.heading = 'Your Account'
this.firstName = 'John'
this.lastName = 'Doe'
this.email = '[email protected]'
}
get fullName() {
// ES6 string interpolation
return `${this.firstName} ${this.lastName}`
}
welcome() {
alert(`Welcome, ${this.fullName}!`)
}
}
<!-- html template -->
<template>
<section>
<!-- one way binding -->
<h2>${heading}</h2>
<!-- event delegation -->
<form role="form" submit.delegate="welcome()">
<div class="form-group">
<label for="fn">First Name</label>
<!-- when using bind Aurelia decides which type of binding to use
in the case of inputs it uses a two-way binding -->
<input type="text" value.bind="firstName" class="form-control" id="fn" placeholder="first name">
</div>
<div class="form-group">
<label for="ln">Last Name</label>
<input type="text" value.bind="lastName" class="form-control" id="ln" placeholder="last name">
</div>
<div class="form-group">
<label for="ln">Email</label>
<input type="text" value.bind="email" class="form-control" id="ln" placeholder="[email protected]">
</div>
<div class="form-group">
<label>Full Name</label>
<p class="help-block">${fullName}</p>
</div>
<button type="submit" class="btn btn-default">Submit</button>
</form>
</section>
</template>
You can check Athena out on the Githubs and the latest version at www.barbarianmeetscoding.com/athena - note that it is yet veeery veeeeery bare yet.
Thoughts on Aurelia So Far
The experience I have had so far with Aurelia has been pretty ok, on one hand I had sort of a hard time setting it up which was a little bit frustrating (I think yeoman has spoiled me in this regard), but on the other hand it was great to write some ES6 and start getting comfy with it. Aurelia has been out in the public barely for a week so I am pretty sure that the aurelia team will soon smooth down the setting up of a project and make aurelia more approachable for everyone.
I haven’t built anything too complex yet so I cannot still be very vocal about how it would be to build a serious application with aurelia, but from my past experience with other frameworks such as Knockout, Durandal or Angular, it feels very nice (cleaner, leaner) to write vanilla javascript in your view models (ES6 modules + classes).
In the upcoming weeks I will continue writing athena and I’ll probably have much more to say about Aurelia. Until then, take care.
Want to Know More?
Here are some references you can take a look if you want to know some more:
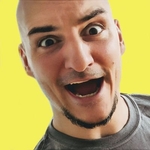
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.