How to Create a Simple Chrome Extension To Hide Your Follower Number On Twitter
Twitter followers.
I know I shouldn’t care, I know I shouldn’t feel bad when someone unfollows me nor overly proud or happy when people follow me but I kind of do.
I suppose it is just human nature and trying to fill that basic need to feel valued and validated… but I will have none of it! Twitter, I shall be driven by intrinsic motivation and not by artificial and arbitrary external rewards!! You shall not control me! (tantrum xD)
Since it seems that I cannot do it by sheer willpower (I have tried), I came up with a better idea. What about removing the source of my troubles completely? Out of sight, out of mind right?
And that’s how this chrome extension and this very blog post came about. Are you curious about how to write a chrome extension to run arbitrary code on any given website? Then follow along!
What are Chrome Extensions?
Chrome extensions are teeny tiny programs that let you extend Chrome’s functionality in ways only limited by your own imagination. You can use the web technologies that you’re familiar with (HTML, CSS and JavaScript) and even interact with any common web API provided by the browser like the DOM API, local storage or geolocation.
How Do You Create a Chrome Extension?
Chrome extensions have a particular structure. They have a manifest.json
file that contains metadata and configuration for the extension itself, and a collection of HTML, JS and CSS files with the actual functionality.
A very simple barebones chrome extension could have a manifest.json
like this one:
{
"manifest_version": 2,
"name": "My extension",
"description": "My extension description",
"version": "1.0",
"browser_action": {
"default_icon": "icon.png",
"default_popup": "popup.html",
"default_title": "Your hovering over me"
}
}
With some metadata for the extension (name
, description
, version
) and some very basic configuration:
- the icon to display on the toolbar (
default_icon
), - a text to show when hovering over the extension (
default_title
) - an html file with markup to display when a user clicks on the extension in the toolbar (
default_popup
).
The popup.html
file could look like this:
<!doctype html>
<html>
<head>
<title>My extension</title>
<style>
body {
font-family: Arial, sans-serif;
font-size: 12px;
}
#status {
width: 600px;
}
</style>
</head>
<body>
<div id="status">This is My Extension!!</div>
</body>
</html>
And would just display a 600px wide box with a text This is My Extension!
.
Ok. Now that we have a basic chrome extension with the amazing functionality of showing a popup and some plain text (Wiho!), the next step is to test whether it works or not.
How Can You Test Whether a Chrome Extension Works?
The easiest way to test whether your extension works or not is to load it into the browser. How would you go about that? Well, you do that by following these steps:
- Open the extension window in Chrome (normally on
More Tools > extensions
or by navigating tochrome://extensions
) - Enable
Developer mode
(yeah, it’s there in the top right corner) - Click on
load unpacked extension
and select the folder in which the extension files are placed (at this point they’d bemanifest.json
andpopup.html
) - The extension should now appear in your Chrome toolbar. If there’s any problem loading the extension you’ll get a comprehensive error message
Go on and try to click on it, the popup with This is My Extension!
should welcome you.
Writing an Extension to Hide Your Number of Twitter Followers
Ok, so let’s get back to the problem at hand, hiding the number of followers on twitter. There’s a special type of CSS and JavaScript files in Chrome extensions that run in the context of existing webpages like twitter. They are called content scripts and can be defined within an extension manifest:
{
"content_scripts": [
{
"matches": ["*://*.twitter.com/*", "*://twitter.com/*"],
"css": ["hide-twitter-followers.css"],
"js": ["hide-twitter-followers.js"]
}
],
"permissions": ["*://*.twitter.com/*", "*://twitter.com/*"]
}
With this addition to the manifest.json
file we map a url or collection of urls *://twitter.com/*
to a javascript hide-twitter-followers.js
and a css hide-twitter-followers.css
files. Essentially we are saying, whenever the user visits any site that matches this pattern *://twitter.com/*
go and run these scripts.
Also notice how we need to add the same url patterns to the permissions
property so that the extension and the user installing it can be aware that this particular extension has access to twitter.
A quick way to test that these files are been loaded is to console.log
something in the javascript file (console.log
for the win!). So we’ll create the hide-twitter-followers.js
file and write the following:
;(function() {
console.log('[HideFollowers] Running hide twitter followers extension')
})()
Now go to the extensions in your Chrome browser and reload the extension (you’ll need to do that everytime you change the manifest). Next visit twitter, open the developer console and you should be able to see the [HideFollowers]
entry.
Content scripts, while having some limitations, give you full access to a websites DOM. So a way to hide twitter followers using javascript could be the following:
;(function() {
console.log('[HideFollowers] Running hide twitter followers extension')
hideFollowers()
function hideFollowers() {
console.log('[HideFollowers] followers')
var stats = document.querySelector('.ProfileCardStats')
if (stats) stats.style.display = 'none'
var statsProfile = document.querySelector(
'.ProfileNav-item.ProfileNav-item--followers'
)
if (statsProfile) statsProfile.style.display = 'none'
}
})()
But a far better approach in this particular case is to just use CSS directly:
.ProfileCardStats .ProfileCardStats-stat:nth-child(2),
.ProfileCardStats .ProfileCardStats-stat:nth-child(3) {
display: none !important;
}
.ProfileNav-item.ProfileNav-item--followers {
display: none !important;
}
If you now go to twitter and refresh the site, then the number of twitter followers should be gone. Bye… for ever!
Releasing an Extension in Chrome Web Store
Packaging your extension and distributing it in the Chrome Web Store is pretty easy. You just go to the Chrome web store developer dashboard, add a new item, fill in the different fields guided by the form and upload your extension bundled within a zip file.
If you are interested in other ways to distribute your extension then take a look at this article on the Chrome Extensions docs.
Where Can I get the Code?
I’ll be uploading the code to GitHub soon (I need to go through an approval process to release open source). In the meantime this is pretty much the full extension code:
// manifest.json
{
"manifest_version": 2,
"name": "Hide Twitter Followers",
"description": "This Extension Hides Your Twitter Followers From Twitter",
"version": "1.2",
"browser_action": {
"default_icon": "icon.png",
"default_popup": "popup.html",
"default_title": "Your twitter followers are hidden"
},
"permissions": ["*://*.twitter.com/*", "*://twitter.com/*"],
"content_scripts": [
{
"matches": ["*://*.twitter.com/*", "*://twitter.com/*"],
"css": ["hide-twitter-followers.css"],
"js": ["hide-twitter-followers.js"]
}
]
}
/* hide-twitter-followers.css*/
.ProfileCardStats .ProfileCardStats-stat:nth-child(2),
.ProfileCardStats .ProfileCardStats-stat:nth-child(3) {
display: none !important;
}
.ProfileNav-item.ProfileNav-item--followers {
display: none !important;
}
// hide-twitter-followers.js
;(function() {
console.log('[HideFollowers] Running hide twitter followers extension')
})()
<!-- popup.html -->
<!doctype html>
<html>
<head>
<title>Hide Twitter Followers PopUp</title>
<style>
body {
font-family: "Segoe UI", "Lucida Grande", Tahoma, sans-serif;
font-size: 100%;
}
#status {
/* avoid an excessively wide status text */
max-width: 600px;
}
</style>
</head>
<body>
<div id="status">Your Twitter Followers Are Hidden</div>
</body>
</html>
Now go forth comrade and build extensions for your own quirky and strange motives! And have yourself a very nice day! :)
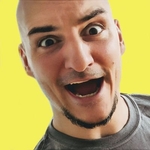
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.