How to Make a VSCode Extension
Over the past few weeks I’ve been working on a VSCode extension to help you learn vim within VSCode. This talk is a summary of some of the things I’ve learned along the way and its goal is to show you how easy it is to get started and encourage you to write your own extensions. Have fun!
And here’s is a transcript version for those of you that aren’t keen on watching videos.
How to create a new VSCode extension
Use yeoman with the vscode extension generator:
npm i -g yo generator-code
Then use that generator to create a new extension:
yo code
This will start a wizard where you can choose different options based on the type of extension that you want to build. Once you’ve completed the wizard you’ll have the skeleton of an extension in a folder of your choosing, for example, my-new-extension
.
Jump inside the extension folder and open VSCode:
cd `my-new-extension` && code .
Now you can start working on your new extension. To give it a try, run F5
or from the command palette use the Start Debugging
command. This will run a new instance of VSCode that has your extension enabled.
If you’ve created a barebones extension you’ll see that it has configured a new command “Hello world” that when executed will show a Hello world toast.
You can find more info at bit.ly/vscode-extension-get-started
The anatomy of a VSCode Extension
package.json
: Your extension manifestsrc
: Your extension’s source code is in thesrc
folderREADME.md
: Your extension’s description. This is what is visile in the VSCode marketplace.CHANGELOG.md
: A list of the versions in your extension and what changes in each version. This is visible from the VSCode marketplace.
VSCode uses package.json
as the manifest for your extension. It extend the normal package.json that you’re familiar with in node.js with some properties that specify in a declarative fashion how your extension enhances VSCode. For example:
contributes
describes how your extension enhances VSCode with command and other functionalityactivationEvents
describes how and when your extension becomes active
All the code for your extension lives inside the src
folder. Within this folder src/extension.ts
represents the entry point to your extension. The extensions.ts
file contains a function that is called to initialize your extension as a response to the activation event and uses VSCode APIs to register commands and enrich VSCode with new functionality.
Development
During development you’ll jump between implementing your extension and interacting with it within VCode:
- Type
F5
or use theStart debugging
command to load a VSCode version with your extension enabled. - When you make changes in the extension and want to see them reflected in VSCode type
Command+R
(orCTRL+R
in Windows) to refresh VSCode and load those changes.
Publish extensions
Once you’re happy with your extension you can package it and share it with your friends, or publish it on the VSCode Marketplace for everyone to enjoy.
You can use the vsce
tool to help you publish your extension. Install via npm
:
npm i -g vsce
And now you can take advantage of the vsce
tool to package and publish extensions:
vsce package
: packages your extension in avsix
file that can be installed in your local VSCode or distributed to your friends (without having to publish it in the marketplace). You can also upload this file to the marketplace via the marketplace UI.vsce publish
: publishes your extension in the VSCode marketplace directly.
Note that in order to publish your extension you need to create a publisher account.
Publishing new versions of the extension
At some point in time you’ll likely need or want to update your extension with bugfixes or new functionality. At that time you’ll want to follow these steps to publish a new version:
- update your changelog with the new updates
- Increment the version in
package.json
- Publish your package with
vcse publish
or, package it withvcse package
and upload it via the VSCode marketplace UI
If you have setup your machine to be able to publish with vcse publish
you can also auto-increment the version
number using that command by providing major
, minor
or patch
following semantic versioning:
$ vsce publish minor
Or using a concrete version number:
$ vsce publish 3.1.0
Automating publishing your extension
Useful resources
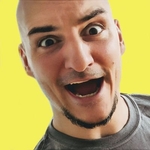
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.