The Basics Of JavaScript Functions
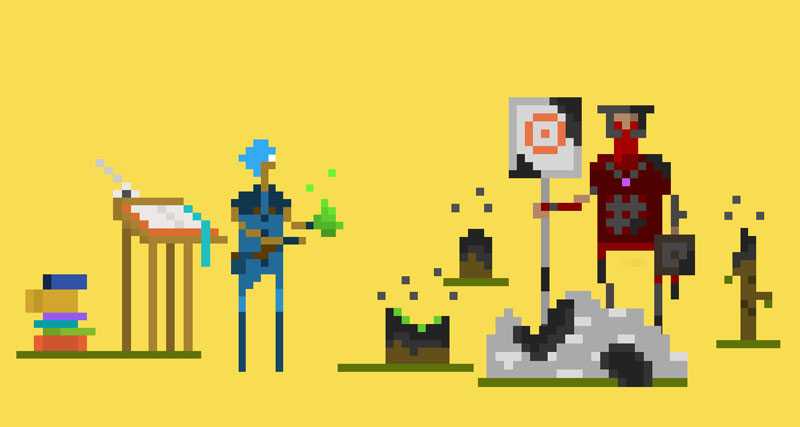
The Mastering the Arcane Art of JavaScript-mancy series are my humble attempt at bringing my love for JavaScript to all other C# developers that haven’t yet discovered how awesome this language and its whole ecosystem are. These articles are excerpts of the super duper awesome JavaScript-Mancy book a compendium of all things JavaScript for C# developers.
Functions are the most foundational building block in JavaScript. Not only do they hold the logic of our applications, they also are the primitives upon which we build other programmatic constructs in JavaScript like classes and modules.
JavaScript provides different ways to declare and use functions, each with their own nuances and limitations, so given that they are such a fundamental part of the language it is important that you are aware of these characteristics when you are writing JavaScript applications.
Welcome to another step in your journey to JavaScript mastery! Let’s get started!
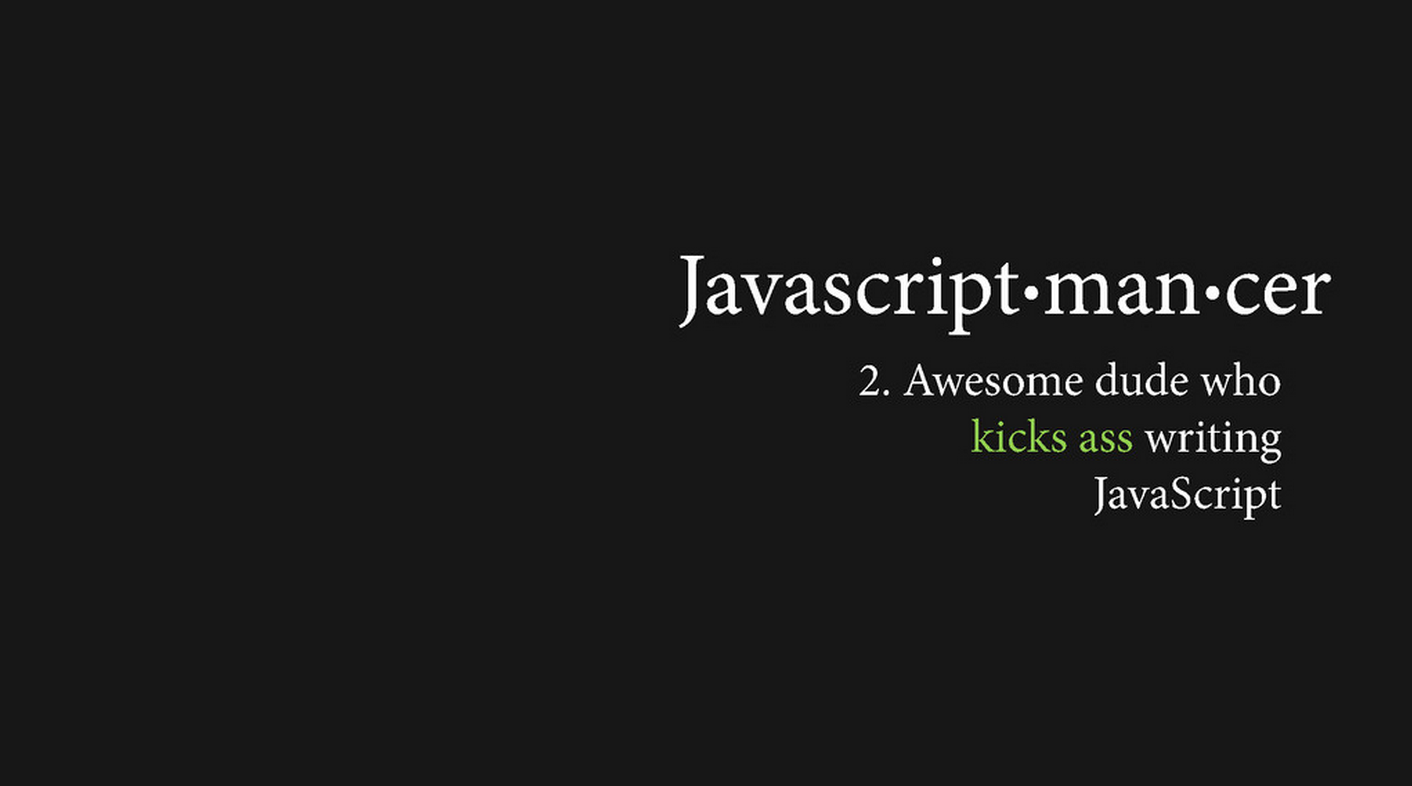
The Basics of Functions
You can experiment with all examples in this blog post within this jsFiddle.
There are two ways to write functions in JavaScript: function expressions and function declarations. It is important to know the implications of using either of these two styles since they work in a very different manner that will impact not only the readability of your code but also how easy/hard it is to debug.
This is a function expression:
// anonymous function expression
var castFireballSpell = function() {
// chaos and mayhem
}
And this is a function declaration:
// function declaration
function castFireballSpell() {
// it's getting hot in here...
}
As you can appreciate, in their most common incarnations function expressions and function declarations look very similar. That’s why it is especially important to understand that they do are different, have different characteristics and behave in different ways from each other.
Let’s examine them in greater detail.
Function Expressions
Function expressions result whenever we declare a function like an expression, either by assigning it to a variable, a property within an object or passing it as an argument to another function (like a lambda):
// an anonymous function expression
var castFireballSpell = function() {
console.log('...chaos and mayhem')
}
// another anonymous function expression as a property of an object
var magician = {
castFireballSpell: function() {
console.log('muahaha! Eat this fireball!')
},
}
// yet another anonymous function expression passed as an argument
var castFireballSpell = function() {
console.log('...chaos and mayhem')
}
There are a couple of important considerations you need to take into account when using function expressions like the ones described above: they are all anonymous functions, and if stored within a variable they are subjected to the same hoisting rules that apply to any other variable.
Anonymous Function Expressions
So even though you read:
var castFireballSpell = function() {
console.log('...chaos and mayhem')
}
and you may be tempted to think that the name of the function is castFireballSpell
, it is not, castFireballSpell
is just a variable we use to store an anonymous function. You can appreciate this anonymity by inspecting the name
property of the function itself:
var castFireballSpell = function() {
console.log('...chaos and mayhem')
}
console.log(castFireballSpell.name)
// => ""
// no name!
Luckily for us, for as long as an anonymous function is bound to a variable the developer tools will use that variable when displaying errors in call stacks (good when debugging):
console.log(
'you can however examine the call stack and see how an anonymous function that is bound to a variable shows the variable name'
)
var castFireballSpellWithError = function() {
console.log('...chaos and mayhem')
try {
throw new Error()
} catch (e) {
console.log('stack: ', e.stack)
}
}
castFireballSpellWithError()
//=> stack: Error
// at castFireballSpellWithError (http://fiddle.jshell.net/_display/:53:15)
// at window.onload (http://fiddle.jshell.net/_display/:58:1)
Even if we use this function as a lambda:
console.log(
'if you use this function as a lambda the name is still shown in the call stack'
)
var functionCaller = function(f) {
f()
}
functionCaller(castFireballSpellWithError)
// => stack: Error
// at castFireballSpellWithError (http://fiddle.jshell.net/_display/:56:15)
// at functionCaller (http://fiddle.jshell.net/_display/:68:35)
// at window.onload (http://fiddle.jshell.net/_display/:69:1)
However, an anonymous function not bound to a variable will show as completely anonymous within the call stack making it harder to debug possible errors (I will call these functions as strict anonymous function in the remainder of the article):
console.log(
"it is only if you use a strict anonymous function that the name doesn't appear in the call stack"
)
functionCaller(function() {
console.log('...chaos and mayhem')
try {
throw new Error()
} catch (e) {
console.log('stack: ', e.stack)
}
})
//=> stack: Error
// at http://fiddle.jshell.net/_display/:76:15
// at functionCaller (http://fiddle.jshell.net/_display/:68:35)
// at window.onload (http://fiddle.jshell.net/_display/:73:1)
This lack of name can also affect the ability to use recursion. Again, as long as we have the anonymous function bound to a variable we can use the closure to access the function and use recursion:
console.log(
'you can use recursion when an anonymous function is bound to a variable'
)
var castManyFireballsSpell = function(n) {
console.log('... SHOOOOOOOOOSH ....')
if (n > 0) castManyFireballsSpell(n - 1)
}
castManyFireballsSpell(3)
// => ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ....
It is important to notice that in this example you are just using a closure to access a variable from within a function, and if you, at some point in time, set the variable castManyFireballsSpell
to another function, you would get a subtle bug where the original function would call this new function instead of itself (so no recursion and weird stuff happening).
A strict anonymous function, on the other hand, has no way to refer to itself and thus you cannot use recursion:
console.log(
"but there's no way for an anonymous function to call itself in any other way"
)
;(function(n) {
console.log('... SHOOOOOOOOOSH ....')
if (n > 0) {
// I cannot call myself... :(
}
})(5)
// => ... SHOOOOOOOOOSH ....
So, in summary, the fact that common function expressions are anonymous affects negatively to their readability, debugging and the user of recursion. Let’s see some ways to ameliorate these issues.
Named Function expressions
You can solve the problem of anonymity that we’ve seen thus far by using named function expressions. You can declare named function expressions by adding a name after the function
keyword:
// named function expression
var castFireballSpell = function castFireballSpell() {
// mayhem and chaos
}
console.log("this function's name is: ", castFireballSpell.name)
// => this function's name is castFireballSpell
A named function expression always appears correctly represented in the call stacks even when used as a lambda (and not bound to a variable):
console.log(
'A named function expression always appears in the call stack, even when used as a lambda not bound to a variable'
)
functionCaller(function spreadChaosAndMayhem() {
console.log('...chaos and mayhem')
try {
throw new Error()
} catch (e) {
console.log('stack: ', e.stack)
}
})
// stack: Error
// at spreadChaosAndMayhem (http://fiddle.jshell.net/_display/:134:15)
// at functionCaller (http://fiddle.jshell.net/_display/:68:35)
// at window.onload (http://fiddle.jshell.net/_display/:131:1)
This helps both while debugging and makes the code more readable - you can read the name of the function and understand what it attempts to do without looking at the specific implementation.
It is important to understand that the name of the function expression is only used internally, that is, you cannot call a function expression by its name from the outside:
var castFireballSpell = function cucumber() {
// cucumber?
}
cucumber()
// => ReferenceError: cucumber is not defined
But you can do it from the function body, which is very useful when working with recursion:
console.log(
'but you can call it from the function body which is helpful for recursioon'
)
;(function fireballSpellWithRecursion(n) {
console.log('... SHOOOOOOOOOSH ....')
if (n > 0) {
fireballSpellWithRecursion(n - 1)
}
})(5)
// => ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ....
// ... SHOOOOOOOOOSH ..... etc..
In summary, named function expressions improve on anonymous function expressions increasing readability, improving the debugging process and allowing for a function to call itself.
Function Expressions are Hoisted as Variables
We still have a problem with hoisting though: Function expressions are hoisted like variables which means that you cannot use a function expression until after you have declared it. This affects readability (since you have to read your code from bottom to top instead of from top to bottom) and can lead to unexpected bugs.
The following example illustrates some of these problems and is also available in jsFiddle:
// if we recap hoisting of variables in JS quickly
// this example:
(function(magic){
magic.enchant2 = enchant;
var enchant = function(){ // something };
}(window.magic || (window.magic = {})))
// is equivalent to:
(function(magic){
var enchant = undefined;
magic.enchant2 = enchant;
enchant = function(){ // something };
}(window.magic || (window.magic = {})))
// you cannot do this with function expressions
(function (magic){
// illustrate how named function expressions help find errors
magic.enchant2 = enchant;
// => hoisting issue, enchant is undefined
// so we are just exposing an undefined variable thinking it is a function
// enchant();
// => TypeError: enchant is not a function
// => hoisting issue, enchant is undefined
var enchant = function enchant(ingredients){
var mixture = mix(ingredients),
dough = work(mixture),
cake = bake(dough);
return cake;
};
// enchant();
// => TypeError: mix is not a function
// hoisting issue, mix is undefined, and so on...
var mix = function mix(ingredients){
console.log('mixin ingredients:', ingredients.join(''));
return 'un-appetizing mixture';
}
var work = function work(mixture){
console.log('working ' + mixture);
return 'yet more un-appetizing dough';
};
var bake = function bake(dough){
oven.open();
oven.placeBaking(dough);
oven.increaseTemperature(200);
// insta-oven!
return oven.claimVictory();
};
var oven = {
open: function(){},
placeBaking: function(){},
increaseTemperature: function(){},
claimVictory: function(){ return 'awesome cake';}
};
}(window.magic || (window.magic = {})));
try{
var cake = magic.enchant2(['flour', 'mandragora', 'dragon', 'chicken foot']);
console.log(cake);
}catch (e){
console.warn('ups!!!!!!', e);
// => ups!!!!!! TypeError: magic.enchant2 is not a function
}
Recap! Both named and anonymous function expressions are hoisted as variables which affects readability and can cause bugs when we try to run a function or expose a function as part of the public API of a module before it has been defined.
Function Declarations
And finally we get to function declarations which have some advantages over function expressions:
- They are named, and you can use that name from the outside and the body of the function itself.
- They are hoisted not as variables but completely together with their definition, this makes them impervious to hoisting problems.
You write function declarations like this:
// function declaration
function castFireballSpell() {
// it's getting hot in here...
}
And they will enable you to write code more similar to the code you are accustomed to write as a C# developer when writing a class, where you declare the public API of a class at the top of the class definition and then you write the implementation of each method from top to bottom, from higher to lower levels of abstraction:
// with function declarations you can write functions like this
// and not worry about hoisting issues
;(function(magic) {
// public API of the magic module
magic.enchant = enchant
// functions from higher to lower level of abstraction
function enchant(ingredients) {
var mixture = mix(ingredients),
dough = work(mixture),
cake = bake(dough)
return cake
}
// these are private functions to this module
function mix(ingredients) {
console.log('mixin ingredients:', ingredients.join(''))
return 'un-appetizing mixture'
}
function work(mixture) {
console.log('working ' + mixture)
return 'yet more un-appetizing dough'
}
function bake(dough) {
oven.open()
oven.placeBaking(dough)
oven.increaseTemperature(200)
// insta-oven!
return oven.claimVictory()
}
var oven = {
open: function() {},
placeBaking: function() {},
increaseTemperature: function() {},
claimVictory: function() {
return 'awesome cake'
},
}
})(window.magic || (window.magic = {}))
var cake = magic.enchant(['flour', 'mandragora', 'dragon', 'chicken foot'])
// => mixin ingredients: flour mandragora dragon chicken foot
// => working un-appetizing mixture
console.log(cake)
// => awesome cake
You can also use function declarations as lambdas (function as value):
var orc = {
toString: function() {
return 'a mighty evil orc'
},
}
var warg = {
toString: function() {
return 'a terrible warg'
},
}
var things = [1, orc, warg, false]
// using the disintegrate function declaration as a lambda
// nice and readable
things.forEach(disintegrate)
function disintegrate(thing) {
console.log(thing + ' suddenly disappears into nothingness...')
}
// => 1 suddenly disappears into nothingness...
// a mighty evil orc suddenly disappears into nothingness...
// a terrible warg suddenly disappears into nothingness...
// false suddenly disappears into nothingness...
Also notice how the following example, although it can look as a function declaration, is actually a named function expression:
things.forEach(function disintegrate(thing) {
console.log(thing + ' suddenly disappears into nothingness...')
})
Concluding: Prefer Function Declarations and Named Function Expressions
Time to recap. Function expressions have some limitations:
- they are anonymous, which can make them less readable, harder to debug and use in recursion, and
- they are hoisted as variables which can lead to bugs and forces you to declare them before you can use them
Named function expressions solve the problem of anonymity, they make your function expressions more readable, easier to debug and enable recursion.
Function declarations solve both the problem of anonymity and hoisting (since they are completely hoisted), and allow you to write code from higher to lower levels of abstraction.
In summary, and based in these characteristics of functions in JavaScript, prefer named function expressions and function declarations over anonymous function expressions.
Coming Next
Hope you have enjoyed this article about the different ways you can write functions in JavaScript. Up next, I will discuss the most common patterns to achieve default values, multiple arguments, function overloading when writing JavaScript functions and I’ll show you how some of the new features in ES 2015 provide native support for some of these.
Interested in Learning More JavaScript? Buy the Book!
Are you a C# Developer interested in learning JavaScript? Then take a look at the JavaScript-mancy book, a complete compendium of JavaScript for C# developers.
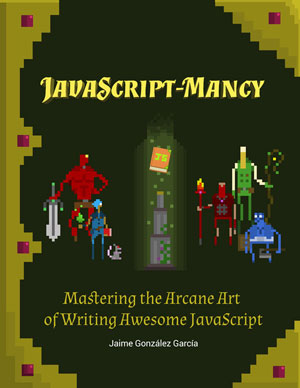
Have a great week ahead!! :)
More Articles In This Series
- Chapter 0: The Basic Ingredients of JavaScriptMancy
- Chapter 1: The Many a One JavaScript Quirks
- Functions
- Object Oriented Programming
- Basics
- ES6
- OOP
- Introduction to OOP in JavaScript for C# Developers
- Summoning Fundamentals: Introduction to OOP In JavaScript – Encapsulation
- Summoning Fundamentals: Introduction to OOP In JavaScript – Inheritance
- Summoning Fundamentals: Introduction to OOP In JavaScript – Polymorphism
- White Tower Summoning: Mimicking C# Classical Inheritance in Javascript
- White Tower Summoning Enhanced: The Marvels of ES6 Classes
- Black Tower Summoning: Object Composition with Mixins
- Safer JavaScript Composition With Traits and Traits.js
- JavaScript Ultra Flexible Object Oriented Programming with Stamps
- Object Oriented JavaScript for C# Programmers
- Tooling
- Data Structures
- Functional Programming
- And more stuff to be shaped out:
- Functional Programming with JavaScript
- Modules
- Asynchronous Programming
- The JavaScript Ecosystem
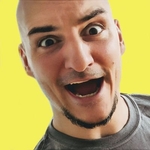
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.