Updating Your Angular 2 App To Use The New Forms API: A Practical Guide
Hi! I have slowly continued to update my Getting Started with Angular 2 Step by Step series from the beta to the latest version of Angular which is now RC4. This time it was the turn of the Forms and Validation article where I used what is known as template driven forms.
Again, just like with the router, there are some changes that you’ll need perform to update your Angular 2 app forms to the latest version, albeit at a much smaller scale. I thought it would be a nice to have these updates in the form of a brief and very practical guide and here we are. Enjoy!
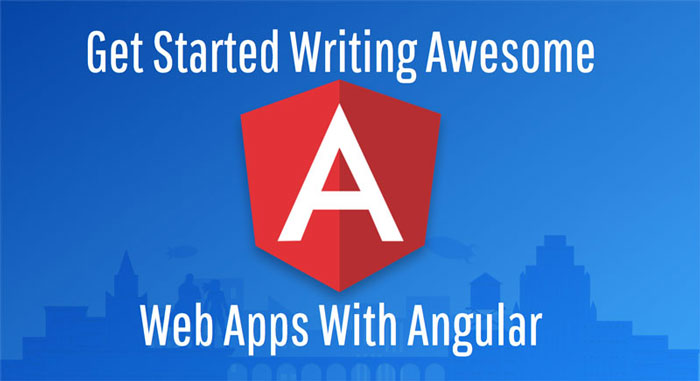
Updating the Angular 2 Forms Step by Step
In order to update your Angular 2 app template driven forms to the latest version you’ll need to follow these steps:
- Enable the new forms API (and disable the old one)
- Remove the
ngControl
directive from your forms - Use
ngModel
instead ofngControl
to gain access to the change tracking and validation features.
1. Enable The New Forms API
In Angular 2 RC2 we got ourselves new forms because of several good points stated right in this change proposal document that you can find here. At present the old forms API is deprecated and only temporary present giving people time to update to the latest version. In order to get access to the new Forms API you’ll need to tweak your application bootstrap slightly:
import { bootstrap } from '@angular/platform-browser-dynamic'
import { disableDeprecatedForms, provideForms } from '@angular/forms'
import { AppComponent } from './app.component'
bootstrap(AppComponent, [
disableDeprecatedForms(), // Disable old Forms API!
provideForms(), // Use new Forms API!
]).catch((err: any) => console.error(err))
See how we are importing two functions disableDeprecatedForms
and provideForms
that let us disabled the old forms API and activate the new one respectively.
Now we can proceed using the new forms API. In future releases on Angular 2 the old forms API will be removed and then you’ll be able to completely forget about this section.
2. Remove the ngControl
Directive
Prior to Angular 2 RC2 we used the ngControl
directive to hook up an input element with Angular 2 forms so that we could enable change tracking and validation for that particular input. An input in an Angular 2 form could look like this:
<label for="name">Name: </label>
<input id="name" name="name" [(ngModel)]="person.name" required ngControl="name">
Where you have ngModel
taking care of two-way data binding and ngControl
providing the change tracking and validation capabilities. The Angular 2 team thought that this approach wasn’t good enough : They wanted to provide a more intuitive and simple API that developers would easily guess and make migrating to Angular 2 easier for Angular 1 developers.
The resulting proposal removes the ngControl
directive in favor of ngModel
. In the new Forms API the input looks like this:
<label for="name">Name: </label>
<input id="name" name="name" [(ngModel)]="person.name" required>
Much better right?
3. Use ngModel
instead of ngControl
In your application you may have some inputs where you want to display validation messages to the user. Prior to RC2 you would achieve this by using ngControl
like this:
<label for="name">Name: </label>
<input id="name" name="name" [(ngModel)]="person.name" required ngControl="name" #name="ngForm">
<div [hidden]="name.valid || name.pristine" class="error">
Name is required
</div>
Where you have the ngControl="name"
enabling change tracking and validation for this particular input, and the #name="ngForm"
that creates a local variable name
that let’s you access the validation information right from the template.
In the new forms API, where there’s no ngControl
anymore we write the equivalent:
<label for="name">Name: </label>
<input id="name" name="name" [(ngModel)]="person.name" required #name="ngModel">
<div [hidden]="name.valid || name.pristine" class="error">
Name is required
</div>
So we remove ngControl
just like we did in the previous section and create a local variable name
by using the expression #name="ngModel"
. This will give us access to the validation API through that name
variable.
And that’s it!
Would you Like To Learn More?
- Angular 2 Forms documentation
- Angular 2 Change Proposal for New Forms. This is a great piece of documentation that argues the reasons from the new forms API and all the breaking changes.
- Angular 2 Forms Cookbook
- Angular 2 ngForm directive documentation
- Template Driven Forms in Angular 2
Have a great weekend!
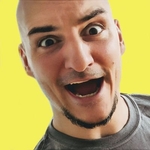
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.