AJAX and XMLHttpRequest
XMLHttpRequest
The XMLHttpRequest
is a web api that allows you to send/receive HTTP requests from the browser to the server. Sending an HTTP request with XMLHttpRequest
consists on these steps:
- Instantiate an
XMLHttpRequest
object - Open a URL
- Optionally configure the
XMLHttpRequest
object and add event handlers for asynchronous HTTP communication. It is better to configure after the XHR is open because some options only work when the XHR is open. - Send the request
var result;
// 1. Instantiate object
var xhr = new XMLHttpRequest();
// 2. open url
xhr.open("get", "http://www.myapi.com/api", /* async */ true);
// 3. configure and add event handlers
xhr.onreadystatechange = function(e){
if (xhr.readyState === 4){ // DONE
if (xhr.status === 200){
result = xhr.response;
} else {
console.error("Error response: ", xhr.status);
}
}
};
xhr.ontimeout = function(e){
console.error("Request timed-out");
}
// 4. send request
xhr.send();
The open
method allows you to provide some basic configuration for the XHR request:
open(method, url, async, user, password);
The send
method lets you send the request with or without data (depending on the type of request):
send();
send(data);
Example: Using XHR to get some JSON
See this jsFiddle:
<button id="get-repos">Get Vintharas Repos! in JSON!!</button>
<pre id="response"></pre>
console.log('loading event handlers');
var $code = document.getElementById("response");
var $getReposBtn = document.getElementById("get-repos");
$getReposBtn.onclick = function(){
var xhr = new XMLHttpRequest();
xhr.timeout = 2000;
xhr.onreadystatechange = function(e){
console.log(this);
if (xhr.readyState === 4){
if (xhr.status === 200){
$code.innerHTML = xhr.response;
} else {
console.error("XHR didn't work: ", xhr.status);
}
}
}
xhr.ontimeout = function (){
console.error("request timedout: ", xhr);
}
xhr.open("get", "https://api.github.com/users/vintharas/repos", /*async*/ true);
xhr.send();
}
XMLHttpRequest properties
Property | Description |
---|---|
readyState | get current state of the XHR object |
response | get the response returned from the server according to responseType |
responseBody | gets the response body as an array of bytes |
responseText | gets the response body as a string |
responseType | gets/sets the data type associated with the response, such as blob, text, array-buffer, json, document, etc. By default it is an empty string that denotes that the response type is string. This is actually only used when deserializing the body of a response, it doesn't affect the HTTP accept headers sent to the server. The way it works is, for instance, that if you set "json", you can get javascript objects directly from the "xhr.response" property, whereas if you leave it empty or use "text" you get a text version of the json response in the "xhr.response" property. |
responseXML | gets the response body as an XML DOM object |
status | gets the HTTP Status code of the response |
statusText | Gets the friendly HTTP status code text |
timeout | Sets the timeout threshold for the requests |
withCredentials | specifies whether the request should include user credentials |
XMLHttpRequest methods
Method | Description |
---|---|
abort | cancel current request |
getAllResponseHeaders | get a complete list of the response headers |
getResponseHeader | get specific response header |
send | make the http request and receive the response |
setRequestHeader | add a HTTP header to the request |
open | set the properties for the request such as URL, username and password |
XMLHttpRequest events
- ontimeout: lets you handle a timeout (having configured the timeout in the XMLTHttpRequest object)
- onreadystatechange: lets you handle the event of the state of the XMLHttpRequest changing within these states:
[UNSENT, OPENED, HEADERS_RECEIVED, LOADING, DONE]
. It is used in async calls. - upload: helps you track an ongoing upload
AJAX and jQuery
jQuery provides simplified wrappers around the XMLHttpRequest
object: $.ajax()
, $.get()
, $.post()
, $.getJSON()
, etc.
$.ajax method
The $.ajax
method allows you to make async HTTP requests:
$.ajax(url [, settings])
$.ajax([settings]) // all settings are optional, you can set defaults via $.ajaxSetup()
A common GET request with $.ajax
could be:
var $response = document.getElementById("response");
$.ajax("https://api.github.com/users/vintharas/repos")
.done(function(data){
$response.innerHTML = JSON.stringify(data,null,2);
}
As you can see the $.ajax
method returns a jqXHR
object with the Promise interface: done
(success), fail
(error), always
(complete) and then
method usually available to promises.
$.get
The $.get
method is a simplification of the $.ajax
method to handle purely get requests:
$.get([settings]);
$.get(url [, data] [, success] [,dataType])
// it is equivalent to
$.ajax({
url: url,
data: data,
success: sucess,
dataType: dataType
})
where:
- url is the url to which the request is sent
- data is an object or string to send to the server
- success is a success callback
- dataType is the type of data expected from the server (xml, json, script or html)
This method also returns a jqXHR
object that acts as a promise.
$.getJSON
The $.getJSON
method is a shorthand for:
$.ajax({
dataType: "json",
url: url,
data: data,
success: success
})
// it looks like this
$.getJSON(url [, data] [, success])
Forms
Sending Data to a server using a Form
Serializing a Form Data With jQuery
References
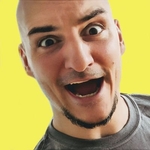
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.