ASP.NET MVC Wiki - ASP.NET MVC 4
This article will focus on ASP.NET MVC 4 the MVC web framework from Microsoft.
- Introduction to ASP.NET MVC 4
- Web Optimization in ASP.NET MVC 4
- Async/Await in ASP.NET MVC 4
- Mobile Web Development and ASP.NET MVC 4
Introduction to ASP.NET MVC 4
Models, Views and Controllers
- Model, View and Controllers
- Controllers
- Action Methods
- Action Results
- Views
- Razor
- Views organized per controller
- Shared views
- Layout templates _Layout
- Partial views and sections
- Controllers
We Application Configuration
- Web app configuration in global.asax and App_Start
- Routing
- Bundling
- Filter
Database Migrations
Create unit of work context that inherits from DbContext
:
// using the IDepartmentDataSource interface allows us
// to avoid having a direct coupling to entity framework
public class DepartmentDb: DbContext, IDepartmentDataSource
{
public DbSet<Employee> Employees {get;set;}
public DbSet<Department> Departments {get;set;}
IQueryable<Employee> IDepartmentDatasource.Employees {get{return Employees;}}
IQueryable<Department> IDepartmentDatasource.Deparments {get{return Deparments;}}
}
You can enable-migrations
in the NuGet Package Manager window. This will create a Migrations folder with a Configuration.cs
file. In this file you can enable automatic migrations (good during development) and provide initial data in your database via the Seed
method:
internal sealed class Configuration: DbMigrationsConfiguration<eManager.Web.Infrastructure.DeparmentDb>
{
public Configuration()
{
AutomaticMigrationsEnabled = true;
}
protected override void Seed(eMaanger.Web.Infrastructure.DepartmentDb context)
{
context.Departments.AddOrUpdate(d => d.Name,
new Department {Name = "Engineering"},
new Department {Name = "Human Resources"},
new Department {Name = "Ninja Ops"},
);
}
}
By default, Entity Framework will go and create a LocalDB database in your App_Data
folder. You can, however, modify this in the DbContext
class by explicitly adding a connection string:
public DepartmentDb(): base("DefaultConnection") // connection string or name of the connection string
{}
You can also use the update-database -verbose
command in the NuGet Package Manager Console to run migrations on the db (and see the actual sql queries).
IoC in ASP.NET MVC
It is a common practice to use IoC containers to manage the instantiation and lifetime of Controllers and of all their dependencies.
Web Optimization in ASP.NET MVC 4
Async/Await in ASP.NET MVC 4
A given web app has a limited number of processing threads. Using these threads to call costly/slow operations in a blocking fashion means that we have less threads available to handle HTTP requests and thus we make some http requests wait before they can be handled.
In MVC 3 we had the possibility of using AsyncController
class. MVC 4 makes it even easier to create asynchronous controllers and action methods using the async\await
paradigm and the Task Paralell library.
Asynchronous Controllers and Actions in ASP.NET MVC 4 look just like synchronous code/controllers/actions decorated with the async
and await
keywords to signal the long-running operations:
public async Task<ActionResult> Index()
{
// code...
model.Weather = await weatherClient.GetCurrentWeatherAsync();
// code...
return View(model);
}
You can run multiple asynchronous tasks in parallel:
await Task.WhenAll(getWeatherTask, getTemperatureTask, getHumidityTask);
You can also specify timeouts for your asynchronous operations (imagine that you make an http request that takes too much time to complete or never completes at all) via the AsyncTimeout
attribute:
[AsyncTimeout(1000)]
public async ViewResult Index()
{
// code
}
Mobile Web Development and ASP.NET MVC 4
References
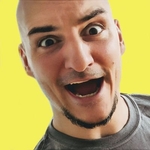
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.