ASP.NET MVC 4 Wiki - The Razor View Engine
Razor is the newest view engine for ASP.NET. It processes ASP.NET views and generates html with dynamic content.
Declaring Strongly Typed Views
In razor, we declare strongly typed views using the @model
identifier:
...
@model Chapter05_Razor.Models.Barbarian
...
So that we can later access the domain object passed to the view via the @Model
identifier, as in:
...
<body>
<div>
Hither came @Model.Name @Model.Description , to tread the jeweled thrones of the Earth under his sandalled feet.
</div>
</body>
which would render:
...
<body>
<div>
Hither came Conan with black-haired, sullen-eyed, sword in hand, a thief, a reaver, a slayer, with gigantic melancholies and gigantic mirth
</div>
</body>
Using Layouts
Layouts in Razor, as in ASPX, are templates that allow code reuse across your web application. A basic layout could look like:
<!DOCTYPE html>
<html>
<head>
<meta name="viewport" content="width=device-width" />
<title>@ViewBag.Title</title>
</head>
<body>
<div>
@RenderBody() <!-- Here we render the razor views that have a layout -->
</div>
</body>
</html>
We can apply a layout to a razor view by assigning the Layout property in its header:
...
@{
ViewBag.Title = "Hello Conan!";
Layout = "~/Views/_BasicLayout.cshtml";
}
...
When a view is self-contained, that is, it contains all the content that we need to render and return to the user, we use the statement below to denote that it does not need a layout:
...
@{
Layout = null;
}
...
Both examples above showcase what we know as Razor code blocks - the blocks delimited by @{...}
- a Razor construct that allows us to include C# statements within the view.
Since it may be cumbersome to set the Layout property in every view, ASP.NET MVC provides a mechanism to set defaults layouts for all views, the _ViewStart.cshtml
file. Whichever content we write in this file will be treated as if it were contained in a view file itself, therefore, we can use this facility to set a default value for the Layout
property.
Razor Expressions
Insert Data Into a View
Just use the classic dot syntax on the @Model
or the @ViewBag
objects.
Set Attribute Values
You can use the same dot syntax to set attributes of html elements.
Use Conditional Statements
Razor is able to process conditional statements as in:
@model Chapter05_Razor.Models.Barbarian
@{
ViewBag.Title = "Player Profile";
}
<table>
<thead>
<tr><th colspan="2">Profile</th></tr>
</thead>
<tbody>
<tr><td>Name</td><td>@Model.Name</td></tr>
<tr><td>Description</td><td>@Model.Description</td></tr>
<tr><td>Weapon</td><td>@Model.Weapon</td></tr>
<tr><td>Armor</td><td>@Model.Armor</td></tr>
<tr>
<td>Status</td>
<td>
@if (@Model.HitPoints >= 75)
{
@Model.Name @: looks perfectly healthy
}
else if (@Model.HitPoints < 75 && @Model.HitPoints >= 25) {
@Model.Name @: looks hurt and walks with difficulty
}
else
{
@Model.Name @: looks nearly dead, he can barely stand and ocassionaly looks into the void, as if seeing something beyond our sight
}
</td>
</tr>
</tbody>
</table>
Notice how we need to use the expression @: some text
when we want to insert string literals into the view that are not contained in HTML elements.
Enumerate Arrays and Collections
You can create a strongly typed view with a collection and use a foreach
loop as usual
...
<ol>
@foreach (var b in @Model)
{
<li>@b.Name</li>
}
</ol>
...
Note About Namespaces
If you don’t want to use full names for referring to classes, you can use @using
expressions to import namespaces as we usually do in C#. For instance
...
@using Chapter05_Razor.Models
...
Note: Sample source code can be found at GitHub.
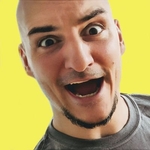
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.