CoffeeScript
CoffeeScript is a beautiful programming language that transpiles to JavaScript and focuses on brevity and readability.
Basic CoffeeScript
Variables
You don’t need to explicitely declare variables in CoffeeScript:
// JavaScript
var message
message = 'Hello World'
alert(message)
// CoffeeScript
message = 'Hello World'
alert(message)
Functions
CoffeeScript uses indentation when defining functions and always assumes that the last expression within your function is going to be returned.
// JavaScript
var hello = function() {
return alert("Hello World");
}
// CoffeeScript
hello = ->
alert("Hello World")
If a given function has parameters you use the notation below:
// JavaScript
var say = function(message) {
return alert(message);
}
// CoffeeScript
hello = (message) ->
alert(message)
Default parameters are expressed via:
// CoffeeScript
hello = (message = "Hello World") ->
alert(message)
// this is the JavaScript that would be generated
var say;
say = function(message) {
if (message == null){
message = "Hello World";
}
return alert(message);
}
Calling functions in CoffeeScript is as easy as:
// CoffeeScript
hello()
say("Hello World")
say "Hello World" // We can remove the parentheses
add(1,2)
add 1 2
Since parentheses can be obviated in every function call, a convention exists to remove the parentheses only in the outermost call to improve clarity. For instance, we would use alert say("hello world")
rather than alert say "hello world"
.
String Manipulation
In CoffeeScript you can use string interpolation to insert variables within a string:
// JavaScript
var many = 10
alert('I drank ' + many + ' beers yesterday')
// CoffeeScript
many = 10
alert('I drank #{many} beers yesterday')
More CoffeeScript Syntactic Sugar
- In CoffeeScript you can use
@
to refer tothis
Installing CoffeeScript
Via Node.js
- Install node.js
- Install npm (node package manager)
- Install CoffeeScript using
npm install -g coffee-script
- Verify it has installed correctly
coffee -h
- To transcompile a CoffeeScript file use
coffee -c test.coffee
- To transcompile a CoffeeScript file every time the source code is updated use
coffee -cw test.coffee
- To transcompile all CoffeeScripts files in a folder use
coffee -c src -o js
- As in 6. you can also use
coffee -cw src -o js
In Visual Studio
CoffeeScript Resources
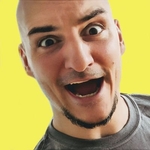
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.