Git Wiki
Help
PS> git help # Access help (list of commands)
PS> git help <commandPS> # Access help for 'command'
Configuration
PS> git config --global user.name "John Doe" # set name of user
PS> git config --global user.email "[email protected]" # user email
PS> git config --global color.ui true # color ui
PS> git config --global core.editor emacs # editor
PS> git config --global merge.tool <difftoolPS> # diff tool
PS> git config user.email "[email protected]" # user email for local repo
PS> git config --list # shows global and local settings
Start a repo
PS> git init # create local repository in the current folder
PS> touch README.md # create README file
PS> git ci -am "Created repository."
Basic commands
PS> git add <filenamePS> # add filename to staging area
PS> git add <list of filesPS> # adds files to staging area
PS> git add --all # add all new, modified or deleted files to the staging area
PS> git add *.txt # add all txt files in the current folder
PS> git add "*.txt" # add all txt files in the whole project
PS> git add . # add all files in this folder and subfolders
PS> git commit -m "message" # commit staged files with message
PS> git commit -am "message" # Add changes from all tracked files and commit
PS> git status # show current status of the repository
PS> git log # shows history of commits
PS> git log --oneline # show all commits in one single line
Note:
- Git uses vi if no default editor is set to edit commit messages
h
: leftk
: upl
: rightj
: downESC
: leave modei
: insert mode:wq
: save and quit (write + quit):q!
: cancel and quit
Diffs
PS> git diff # show unstaged differences since last commit
PS> git diff HEAD # like git diff
PS> git diff HEAD^ # git diff parent of last commit against current
PS> git diff HEAD^^ # git diff grandparent of last commit against current
PS> git diff HEAD^5 # git diff 5 commits ago against current
PS> git diff HEAD^..HEAD # show diff of second most recent commit against the most recent
PS> git diff --staged # show staged differences since last commit
PS> git diff <SHA1PS> <SHA2PS> # show diff between commits identified by SHA's or abbreviated SHA's
PS> git diff <branch1PS> <branch2PS> # do a diff of two branches
PS> git diff --since=1.week.ago --until=1.minute.ago # You can use time based ranges to do diffs
Unstage, reset or cancel
PS> git reset HEAD <filePS> # Unstage file
PS> git checkout -- <filePS> # Reset file to state in last commit
PS> git reset --soft HEAD^ # Undo last commit and put changes in the staging area
PS> git reset --hard HEAD^ # Undo last commit completely
PS> git reset --hard HEAD^^ # Undo last two commits completely
Notes:
- HEAD refers to the last commit on the current branch
- HEAD^ refers to the second last commit on the current branch
- HEAD^^ refers to the third last commit on the current branch
- It is recommended not to undo commits once you have pushed changes to the canonical repository
Ammend a commit
PS> git commit -ammend -m "message" # Adds staged files to last commit. The commit message will override the previous one
Remote repositories. Pushing and pulling
PS> git remote add origin <remote repoPS> # by convention use origin for canonical remote
PS> git remote -v # show remote repositories (verbose)
PS> git remote rm <namePS> # remove remote repositories
PS> git push -u <remotePS> <branchPS> # push local repository to remote one
PS> git pull <remotePS> <branchPS> # pull changes from remote to local repository
Note:
- The -u option sets the default upstream repo so you can use “git push” and “git pull” without specifying a remote
Cloning a repository
PS> git clone <addressPS> # clone repository into local repo named as the repo
PS> git clone <addressPS> <namePS> # clone repository into local folder <namePS>
Branches
PS> git branch # list local branches
PS> git branch <branch namePS> # Create a new branch
PS> git branch -d <branch namePS> # Delete a branch
PS> git branch -D <branch namePS> # Force delete a branch (the normal -d will result in a warning if there are changes in the branch that haven't been merged anywhere)
PS> git checkout <branch namePS> # Switch to a branch
PS> git checkout -b <branch namePS> # Create a new branch and switch to it
PS> git push origin <branch namePS> # Push branch to remote branch
PS> git push origin <local branch namePS>:<remote branch namePS> # Push local branch to the remote branch that we select
PS> git merge <from branchPS> # merge a branch to the current branch
PS> git branch -r # list all remote branches
PS> git push origin --delete <branch namePS> # Delete a branch in the remote "origin" repository
PS> git push origin :<branch namePS> # Delete a branch in the remote repository
PS> git push origin <branch namePS> # Push branch to remote branch (this also works with newly created local branches)
PS> git remote show origin # shows remote branches and how they map to local branches
PS> git remote prune origin # clean up references to stale remote branches (remote branches that have been deleted)
Tagging
A tag is a reference to a specific commit.
PS> git tag # List tags
PS> git checkout v0.0.0.1 # Check out code at commit tagged v0.0.0.1
PS> git tag -a v0.0.0.1 -m "version 0.0.0.1" # Add a new tack
PS> git push --tags # Push tags to a remote
Rebase
Rebasing allows you to have a better commit history by removing the necessity of merging branches via the merge
command and thus the existence of merge commits.
A rebase consists on two steps:
-
Fetch changes for remote repository
-
Rebase
- Move all changes to master which are not in origin/master to a temporary area
- Run all origin/master commits
- Run all commits in the temporary area, one at a time
PS> git fetch # fetch
PS> git rebase <branch namePS> # rebases from branch
If you want to, for instance merge a local branch to master via rebase you would:
- Go to feature branch:
git checkout feature-101
- Rebase from master:
git rebase master
- Go to master and merge
git checkout master
git merge feature-101
When there are conflicts during rebase, git will prompt us to resolve the conflict and run the rebase command with different arguments based on if we want to continue, skip the conflicting commit, or abort the rebase.
PS> git rebase --continue # Use to continue rebasing after resolving conflict
PS> git rebase --skip # Use to skip the conflicting commit
PS> git rebase --abort # Use to checkout the original branch and stop rebasing
History
PS> git log # show branch history
PS> git config --global color.ui true # colorize ui and thus log as well
PS> git log --pretty=oneline # show history as one line commits
PS> git log --oneline
PS> git log --pretty=format:"%h %ad- %s [%an]" # this allows you to customize the format of the log
PS> git log --oneline -p # Show what changed in each commit (files and file contents) (patch output)
PS> git log --oneline --stat # Show how many inserts and deletions per file and commit
PS> git log --oneline --graph # Visual representation of branches and commits
PS> git log --until=1.minute.ago # use dates to limit log command
PS> git log --since=1.day.ago
PS> git log --since=1.hour.ago
PS> git log --since=1.month.ago --until=2.weeks.ago
PS> git log --since=2010.10.10 --until=2012.12.12
Note: Some of the options you can use in git log --pretty:format
are:
%h
SHA hash%ad
author date%s
subject%an
author name%d
ref names
run git help log
for more options.
Blame
You can use the blame
command to see all the changes (line by line) on a given file throughout the whole file history and who made those changes.
PS> git blame index.html --date short # show all changes in index.html and who made them
Excluding Files
You can exclude files in your local repository by putting them in the .git/info/exclude
file, either specifically or using patterns.
You can ignore files completely and in all repositories by adding files and file patterns to the .gitignore
file in your repository.
Removing files
PS> git rm <file namePS> # remove file
Untracking file
To untrack a file (remove it from being tracked by git but not from your actual file system) use:
PS> git rm --cached <file namePS> # untrack file
Useful Aliases
These are some useful git aliases:
PS> git config --global alias.co checkout
PS> git config --global alias.br branch
PS> git config --global alias.ci commit
PS> git config --global alias.st status
PS> git config --global alias.mylog "log --pretty:format..."
PS> git config --global alias.lol "log --graph --decorate --pretty=oneline --abbrev-commit --all"
Resources
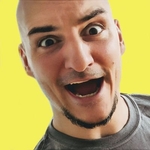
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.