functional-programming
This article is part of my personal wiki where I write personal notes while I am learning new technologies. You are welcome to use it for your own learning!
Achieve Linear Dataflow with Container Style Types
Imagine you have this imperative style code:
const nextCharFornumberString = str => {
const trimmed = str.trim();
const number = parseInt(trimmed);
const nextNumber = number + 1;
return String.fromCharCore();
}
const result = nextCharFornumberString(' 64 ');
console.log(result);
// => 'A'
You can refactor to one single data flow instead of separate lines and state by wrapping it in an array and taking advantage of Array.prototype
functions:
const nextCharFornumberString = str =>
[str]
.map(s => s.trimg())
.map(s => s.parseInt(s))
.map(i => i + 1)
.map(i => String.fromCharCode(i));
const result = nextCharFornumberString(' 64 ');
console.log(result);
// => ['A']
Now we have a single linear data flow. Each assignment that we had before is now captured inside its own isolated context (the arrow function), each expression has its own state completely contained inside that context.
You can even take it one step further and create your own type to represent this isolated context: Box
(which happens to be the Identity Functor)
const Box = x => ({
map: f => Box(f(x)), // fluent API
inspect: f => `Box(${x})`,
fold: f => f(x)
})
Now you can rewrite the previous code:
const nextCharFornumberString = str =>
Box(str)
.map(s => s.trimg())
.map(s => s.parseInt(s))
.map(i => i + 1)
.map(i => String.fromCharCode(i));
const result = nextCharFornumberString(' 64 ');
console.log(result);
// => Box('A')
// You can extract the original value with fold
console.log(result.fold());
// => 'A'
References
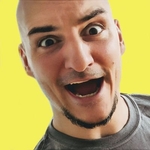
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.