Wiki: React.js
This article is part of my personal wiki where I write personal notes while I am learning new technologies. You are welcome to use it for your own learning!
React.js is a javascript library developed at Facebook to build interactive UIs that focuses on the View part of MVC.
Everything within a React is a component. A component has a state (mutable) and properties (immutable) and produces some arbitrary HTML code.
React provides a special language called JSX, a javascript-meets-html-like language that let’s you embed html templates into javascript code in a very straight-forward manner. jsx
files are compiled into javascript and result in building a virtual DOM. Adding this additional layer of abstraction between and application and the real DOM, provides React with a lot of flexibility to improve the performance tied to DOM manipulation.
A Simple React JS Component
A simple example of a react component written in jsx
could be this button:
// Create new component
var Button = React.createClass({
render: function() {
return <button>Hello World</button>
},
})
You can create a simple component by using the React.createClass
method and passing an object that contains a render
method which returns a JSX template of how the component is going to look like when rendered. You can render the component in the DOM by using React.createElement
followed by React.render
:
// render component
var element = React.createElement(Button)
// add element to the dom
React.render(element, document.body)
Components Can Have Properties
A react component can have properties that can be displayed in the rendered HTML template. You can pass these properties as a vanilla JavaScript object in the React.createElement
method and access them inside the React component via the this.props
property. React lets you inject these properties inside the HTML template by using curly braces {this.props.messages}
. For instance, this component:
// define a component
var Badge = React.createClass({
render: function() {
return (
<button className="btn btn-primary">
{this.props.title} <strong>{this.props.number}</strong>
</button>
)
},
})
// render component
var element = React.createElement(Badge, { title: 'Inbox', number: 5 })
// add element to the dom
React.render(element, document.body)
Would render an HTML like this:
<button class="btn btn-primary">Inbox <strong>5</strong></button>
Nesting Components
Once you have defined a series of components you can easily nest them inside your JSX files. For instance, if you have a navigation item you can nest the previous Badge
component in a very straightforward fashion:
var Nav = React.createClass({
render: function() {
return (
<nav>
<ul>
<li>
<Badge title={this.props.title} number={this.props.number} />
</li>
</ul>
</nav>
)
},
})
// render component
var element = React.createElement(Nav, { title: 'Inbox', messages: 5 })
// add element to the dom
React.render(element, document.body)
In this example the Nav
component includes a nested Badge
component. When we render the Nav
element we pass an options object whose title
and number
will be in turn passed to the Badge
component via its attributes.
Here is another example with a card that contains an image and a badge:
var Card = React.createClass({
render: function() {
return (
<div className="thumbnail">
<img src={this.props.cardImageUrl} />
<div className="caption">
<h3>{this.props.header}</h3>
<p>{this.props.description}</p>
<p>
<Badge title={this.props.title} />
</p>
</div>
</div>
)
},
})
var card = React.createElement(Card, {
title: 'Sing up',
cardImageUrl: 'https://facebook.github.io/react/img/logo.svg',
header: 'awesome',
description: 'jajajajrajjjafdasf',
})
// add component to the DOM
React.render(card, document.querySelector('.root'))
References
- React.js website
- ReactJS: Getting Started at Pluralsight
- Build Web Apps with ReactJS and Flux at Udemy
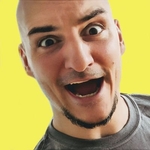
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.