LESS
LESS is a CSS pre-processor that attempts to alleviate the limitations of CSS:
- Prone to code duplication since there are no structures that allow code reuse like variables, classes, etc
- More prone to errors. For instance, if we want to change color scheme we may need to change the same color in different places.
- Lack of functions or ways to encapsulate and execute calculations
- Deep cascading rules make it very hard to debug CSS and know where rules come from (mitigated somehow by modern browser)
- Hard to scale
- Problems with importing many css files, no support for asset handling
- Easy to write bad, unmaintainable CSS
- Easy to write erroneous CSS
By introducing:
- variables to store css rules (like colors)
- reusable components of CSS
- improve the clarity of how rules cascade in CSS
- allow calculations in CSS
- support for asset handling
What is LESS
LESS defines itself as a Dynamic Style Sheet Language, that transcompiles to CSS. It introduces programming features to CSS but it is just a superset of CSS, that is, CSS is valid LESS.
Use LESS on the Browser
You can use less directly in the browser by linking a LESS stylesheet using the rel="stylesheet/less"
attribute and adding the less.js
library to your web page as illustrated below:
<html>
<head>
<link ref="stylesheet/less" type="text/css" href="css/styles.less">
<!-- needs to be after the less stylessheets that need to be processed -->
<script src="js/less.js" type="text/javascript"></script>
</head>
...
</html>
This is not recommended in a production scenario since it involves an additional pre-processing stage when loading your web in the browser. It is much preferred to do this kind of pre-processing in the server and just serve pure CSS to the browser.
Use LESS in the Server
.NET and Visual Studio
You can easily integrate LESS into your development process in .NET and Visual Studio by installing it via NuGet:
PS>> Install-Package dotless
Once installed, all your less
file will be automatically processed into CSS
files. If you take a look at your Web.config
you will be able to appreciate a new section called dotless
that will contain the configuration to pre-process your less
files (caching, minimizing, etc).
Node.js
If you are interested in using LESS in node.js, take a look at the less npm package or yeoman.
LESS Basics
// import in less embedds less files into
// one single css file as opposed to making
// an additional roundtrip to get the extra
// css files
@import "morestyles"
@import "somemorestyles.less"
// You can easily declare variables in less by
// using this syntax
@baseFontSize: 12px;
@accentColor: blue;
// This is a single line comment like in C#
/* This is a normal CSS comment */
// we can nest rules
// which saves us from writing a lot of code
// and also help us visualize the CSS rules
#content
{
h1
{
color: @accentColor;
}
p
{
font-size: @baseFontSize;
// margin: 20px; It is commented out!!
}
}
Variables
Variables are defined by appending a @
symbol to a word and are:
- Case sensitive
- Constant and immutable
- They can be used to store
- Colors
- Units
- strings
- complex types (like a short hand font rules)
@thecolor: #fff;
@somePixels: 12px;
@andSomeEms: 1em;
@strings: Helvetica, Raleway, sans-serif
@awesomeBorder: 2px #eee solid 0 0;
Operations and Functions
You can perform calculations or operations as you would expect of any programming language (even with colors), for instance:
font-size: 12px + 4;
font-size: @baseSize + 4;
It also provides very helpful functions to operate on colors:
// note that you need to use numerical color values
// to be able to apply these color functions
color: ligthen(@color, 25%);
color: darken(@color, 25%);
color: saturate(@color, 25%);
color: desaturate(@color, 25%);
color: fadeIn(@color, 25%);
color: fadeOut(@color, 25%);
color: fade(@color, 25%);
color: spin(@color, 25%);
color: mix(@color, @anotherColor);
and functions to obtain information from colors:
@hue: hue(@color);
@sat: saturation(@color);
@ligt: lightness(@color);
@alpha: alpha(@color);
@color: hsl(10%, 10%, 10%);
and basic mathematical functions such as round
, ceil
, floor
and percentage
.
Mixins
Mix-ins provide support for repeatable sections of CSS. They feel like functions because the take input arguments. They can have default arguments, overloads and can apply values to multiple rules.
// this adds rounded corners to all browsers
// and it has a default value of 5px
.rounded-corners (@radius: 5px) {
-webkit-border-radius: @radius;
-moz-border-radius: @radius;
-ms-border-radius: @radius;
-o-border-radius: @radius;
border-radius: @radius;
}
#header {
.rounded-corners;
}
#footer {
.rounded-corners(10px);
}
Nested rules
Other Features
References
- A Better CSS: LESS and SASS at Pluralsight
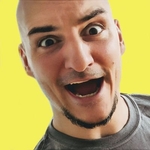
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.