Lua
What is Lua?
Lua is a lightweight, high-level, multi-paradigm programming language with a minimalistic and easy to learn syntax.
Installing Lua
brew install lua luarocks
Once installed you can run the lua repl by writing lua
in your terminal:
▶ lua
Lua 5.4.3 Copyright (C) 1994-2021 Lua.org, PUC-Rio
>
Or interpret a lua script running the lua interpreter:
lua myprogram.lua
Variables
You can create variables in Lua by assignment a value to a variable like so:
isGood = true
number = 42
number2 = 12.23
str = 'a string'
str2 = "another string"
str3 = --[[
A multiline string
that expands
multiple lines.
--]]
Variables are global by default, you can create a local variable using the local
keyword:
number = 42 -- global variable
local number = 42 -- local variable (block scope)
Comments
-- This is a comment in lua
--[[
This is a multiline comment in lua
--]]
Control Flow
-- if/else
if age > 21 then
print('Allowed to drink beer! Yippie!')
elseif age > 20 then
print('Almost there')
else
print('Not allowed to drink beer')
end
-- while loop
while countdown > 0 do
countdown = countdown - 1
end
-- for loops
sum = 0
for i = 1, 100 do -- Range includes both ends.
sum = sum + i
end
-- Count down
sum = 0
for i = 100, 1, -1 do -- Range: begin, end[, step]
sum = sum + i
end
-- Another loop construct:
repeat
print('the way of the future')
num = num - 1
until num == 0
-- Iterating over a table
-- pairs -> iterates over key/values, order not guaranteed
for key,value in pairs(table) do
print(key, value)
end
-- Iterating over an array
-- ipairs -> iterates over numeric keys, order guaranteed
for index,value in ipairs(array) do
print(index, value)
end
-- you can ignore keys
for _,value in ipairs(array) do
print(value)
end
Logic operators
== -- equal
~= -- unequal
-- Undefined variables return nil.
-- This is not an error:
foo = anUnknownVariable -- Now foo = nil.
-- Only nil and false are falsy; 0 and '' are true!
if not foo then print('it was false') end
if not false then print('it was false') end
if 0 then print('it was true') end
if {} then print('it was true') end
if '' then print('it was true') end
-- 'or' and 'and' are short-circuited.
-- This is similar to the a?b:c operator in JS:
result = false and 'yes' or 'no' --> 'no'
Input and Output
print("hello world");
io.write("hello world") -- Defaults to stdout
Tables
Tables are lua’s unique compound data structure, they can be used to describe objects (dictionaries) or arrays.
-- a table literal defines key/value pairs
person = { height = 180, weight = 91}
-- index notation lets you access values
print(person.height) -- 180
person.weigth = nil -- removes a key from the table
-- you can have non-strings as keys as well
table = {[12] = "jaime", [22] = "jamie", [{}] = "wop"}
-- when you call a function with one single param, and that
-- param is a table you don't need to use parentheses. This
-- kind of looks weird, but is a very common pattern in lua:
print{name="jaime", height=180}
-- table iteration
for key, value in pairs(table) do
print(key, value)
end
-- _G is a special table of all globals.
print(_G['_G'] == _G)
-- You can use tables as an array if you don't specify a key:
people = {"john", "jane", "jaime"}
-- list iteration (note how indices start at 1)
for i = 1, #people do -- #people is the size of the list
print(people[i])
end
-- table operations
table.insert(myTable, value) -- adds value to table
Error handling
-- Imagine a function that throws an error when it tries to access something
-- which doesn't exist e.g. this function will throw an error if we send nil as
-- table
function printField(table, field)
print(table[field])
end
local person = nil
-- you can use a pcall to handle errors in lua
-- pcall means protected call and lets you handle errors smoothly:
local ok, result = pcall(printHeight, person, "height")
-- More info: https://www.lua.org/pil/8.4.html
Resources
- lua.org
- Lua live demo environment
- Learn Lua in Y minutes
- luarocks, the lua package manager.
- Books
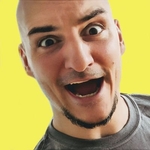
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.