Unix Basics
This article is part of my personal wiki where I write personal notes while I am learning new technologies. You are welcome to use it for your own learning!
Basic Commands
Files and Directories
- List files
ls
- List files with inode numbers
ls -i
- List all
ls -a
- List in long format (more details0
ls -l
- Mark directories with a trailing slash and executable files with a trailing asterisk
ls -F
- Recursively lists subdirectories encountered
ls -R
- Gives the size of each file
ls -s
- Sorts by time modified instead of by name
ls -t
- List files with inode numbers
- Copy file
cp
(cp file1.txt file2.txt
) creates a duplicate- Copy directories
cp -r
as in recursively (cp -r cookies cookies2
) - The interactive flag prompts you whether you want to overwrite a file or not
cp -i
- Copy directories
- Move file
mv
(mv file1.txt file.txt
)- Also interactive with
mv -i
- Don’t use wildcards
*
with mv unless you move to a directory. Themv
command doesn’t know what to do if you specify a target file name, it will just put them all on top of each other
- Also interactive with
- Remove file
rm
(rm file1.txt
)- Remove directories
rm -r
- Remove directories
- Print text in command line
echo
(echo hello wold
) - Redirect output to file
>
(echo hello world > file.txt
) - Create link
ln
(ln file.txt link.txt
). Links to the same index node (inode) that other file. - Create symbolic (soft) link
ln -s
(ln -s file.txt slink.txt
). Links to a filename. Useful when linking files between different filesystems.- You can only create symbolic links to folders
ln -s
(ln -s cookies cookies3
)
- You can only create symbolic links to folders
- Manual
man
(man ls
) alsoman [section] name
(man 5 ls
)- If you don’t know the name of the command exactly you can use
apropos
(apropos copy
will search all headlines for the word copy).man -k
does the same asapropos
- If you don’t know the name of the command exactly you can use
- Catenate
cat
- Print file contents
cat file.txt
- Print multiple file contents
cat file.txt file2.txt
- Write files to another file (catenating)
cat file.txt file2.txt > files.txt
- Print file contents
- Display in a paged manner with
more
- See contents of a file
more filename
- scroll entire screen with
spacebar
, a line withENTER
- enter
vi
by typingv
- quit reading with
q
- scroll entire screen with
- Display the output of other program in a paged manner with
|
likecommand | more
- See contents of a file
- Print working directory
pwd
- Create directory
mkdir
(mkdir cookies
)- Remove directory
rmdir
(rmdir cookies
)
- Remove directory
- Change directory
cd
(cd cookies
). Current directory.
. Parent directory..
.
Paths
- Relative paths
lala/lala/lala
(start in the directory you are in) - Absolute paths
/lala/lala/lala
(start in the root directory) - Wildcards
*
- list all txt files
ls *.txt
- list all txt files that start with cheese
ls cheese*.txt
- list all txt files in all subdirectories of current dir
ls */*.txt
- list all txt files
- If you have spaces in your path,
- use quotation marks
cd "john doe"
- escape it with a backslash
ls john\ doe
- use quotation marks
Wildcars in the Shell
*
match any number of characters in a filename or directory, including none?
match any single character[]
enclose a series of characters that can be matched at a specific position-
within[]
denotes a range of characters~
at the beginning of a word expands to the name of your home directory. If you append a username to it, then it’s that user’s home directory.- Redirecting input/output
<
send input to a command>
create a file and send output to it>>
append output to an existing file
- Pipelining
- pipes are a convenient way to channel the output of a command to the input of another command without creating an intermediate file
ps -aux | sort | more
would give you a paginated alphabetical list of all running processes- note that if a command cannot read from standard input then it cannot be put to the left of a pipe
- Repeat commands from history
!!
repeat last command!git
repeat most recent command that starts withgit
?status
repeat most recent comand that containsstatus
Deleting Stuff
- Delete world
CTRL+w
- Delete line
CTRL+u
Control Terminal Output and Programs
- Suspend output
CTRL+s
- Abort program
CTRL+c
- Discard output
CTRL+o
until anotherCTRL+o
is typed - Susped program
CTRL+z
- See all current running programs
jobs
- Resume suspended program in the foreground with
fg
and in the background withbg
User Session
- Log in remotely
rlogin
- Change passwrod with
passwd
- Exit
exit
orCTRL+d
(useset ignoreof
to avoid exiting by mistake when you typeCTRL+d
)
Permissions
- find out which user you are logged in as
whoami
- find out which gorup you belong to
groups
- execute a file
- unless a file is part of your path you need to prepend the path to it to run it
./file.sh
- unless a file is part of your path you need to prepend the path to it to run it
- Display permissions through
ls -l
- Change permissions using
chmod
- change user permisions
chmod u+rwx file.txt
(add rwx to user) - change group permissions
chmod g+rwx file.txt
(add rwx to group) - change others permissions
chmod o+rwx file.txt
(add rwx to others) - you can combine them at once
chmod ugo+rwx file.txt
- use a
-
sign to remove permissions - you can also use numbers from
0
(no permissions) to7
(all permissions) f.i.chmod 777
(all permissions for user, group and others).755
and754
are common combinations (restrict write to groups and others, restrict write to groups and write,execute to others)
- change user permisions
More Useful Commands
alias
lets you create shorcuts. For instance,alias rm 'rm -i'
. UNIX substitutes command 1 with command 2 when you type it in the terminal- You can remove an alias with
unalias
- You can remove an alias with
ap
magic autopilot commandcompress
anduncompress
compresses using adaptive Lempel-Ziv algorithm (pack
unpack
also compresses using Huffman)df
shows the space free on diskdiff
lets you diff two files.diff -b
ignores white spacediff -i
removes case sensitivity
du
provides disk usage informationecho
echoes a string into the terminal. Useful to see the content of environment variables (echo $TERM
orecho $PATH
)find
find files recursively in a directory matching a logical expressionfinger
shows information about users. It can be used locally and across the internetsftp
to copy files across the networkgrep
(get regular expression) searches for a expression in a file or group of files.grep
expands wildcard characters in the given expressionegrep
(extended grep) searches the expression including alternations (usually faster than grep/fgrep)fgrep
(fixed-string grep) only searches for the string and doesn’t expand wildcards- Wildcard characters
\\
: escape wildcard character after\\
[]
: match any character within brackets (grep [cm]an *.js
).
: match any character (grep eg. *.js
)^
: match beginning of line$
: match end of line*
: match 0 or more of the preceding character
- Useful options
-f
matches all the expressions in a given file as opposed to the one typed in the command line-i
removes case sensitivity-n
displays the line numbers containing a match-l
displays the names of the files that contain a match but not the lines that contained a match-v
displays lines that don’t match
- Examples
- Find all javascript files with variable
foo
:egrep foo *.js
(add the option-n
to get line numbers)
- Find all javascript files with variable
history
displays a list of the commands you’ve previously typed (you must have configured it viaset history=n
wheren
is the number of commands to save)kill
kills a program (f.i.kill -9 processid
useps
to retrieve theprocessid
of a program)- if the process was started by the current cli you can use
jobs
to get the process index andkill -9 %n
- if the process was started by the current cli you can use
look
uses the system dictionary to search for a word. Useful for correcting spelling- you can also use
spell
to check spelling
- you can also use
ps
(process status) shows the status of currently running processesscript
records everything you type in a terminal and every that is outputed within a file (script logsession.txt
)setenv
lets you set environment variables (setenv CUCUMBER 200
)source
sends the contents of a file into the UNIX shell.- If you have a series of aliases that you usually use, you can use
source .myaliases
instead of typing them yourself
- If you have a series of aliases that you usually use, you can use
tar
(tape archiver) lets you store a bunch of files in a single file. (tar key name
)- c Creates a new tape
- f Used for taring to a tape
- t Lists the contents of a tar file
- v Turns verification on
- x Extracts selected files. If no file argument is given the entire contents of the tar file is extracted
- For instance
- create tar file
tar cfv mysourcedir/files directory
- extract tar file
tar xfv mysourcedir/files directory
- create tar file
- For more stuff go to UNIX is a 4 letter word
References
- The Linux Command line
- UNIX is a 4 letter word
- Code School Unix Basics Part I and Part II
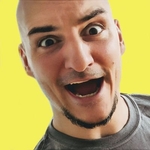
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.