HTML5 Web Sockets
The web sockets API allows to establish socket connections between a browser and a server. As opposed of the stateless, disconnected, common HTTP request/response cycle in which a client communicates with the server, web sockets allows for a persistent connection in which any of the parties, client or server, can send data to the other at any time. (Really useful for real time web applications where the server sends data as it appears to the client).
The core of the API is the WebSocket
object. You can create a new web socket as illustrated below:
// web socket echo server
// useful for testing web sockets
// note the ws:// that represents the URL schema
var connection = new WebSocket('ws://echo.websocket.org');
// you can also use a secure connection using wss://
var connection = new WebSocket('wss://echo.websocket.org');
// and you can also specify subprotocols
var connection = new WebSocket('wss://echo.websocket.org', ['soap', 'xmpp']);
In order to be able to be able to handle messages over web sockets you need to handle some events:
connection.onopen = function() {
connection.send('hello! The connection is open');
}
connection.onerror = function(error){
console.error('Houston we\'ve got a problem');
}
connection.onmessage = function(e){
console.log('Message received from server: ', e.data);
};
You can find an example of web sockets in JsFiddle.
References
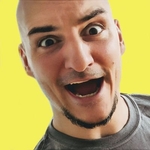
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.