Zsh, Oh-my-zsh and the shell
Zsh is an alternative unix shell to the more common bash.
Table of Contents
File system
$ pwd # print working directory
$ cd . # go to current directory
$ cd .. # go to parent directory
$ cd - # go to last directory
$ cd ~ # go to home directory
$ cd # go to home directory
$ mkdir something # create directory
$ mkdir something/some/thing -p # creates intermediate directories too
Redirecting input and output
Linux programs have built-in support for reading from stdin, producing output to stdout and sending errors to stderr. By default, stdin comes from the keyboard while both stdout and stder are sent to the terminal.
Inputs and outputs can be manipulated using redirection commands:
# > redirects stdout to a file
# This writes the contents of vimrc in file.txt
# It empties the contents of file.txt and overwrites it
$ cat vimrc > file.txt
# >> redirects stdout to a file and appends to it
$ cat vimrc >> file.txt
Pipes
Unix programs can work on their own, but many of them are also designed to behave like building blocks that can be combined with other programs to fulfill more complex tasks (than they can on their own). You can combine unix programs together using pipelines or pipes for short with the pipe operator |
.
How does this work? Most unix programs can consume information from the standard input stdin
and produce information to standard output stdout
. The pipe operator lets you pipe the stdout
of a program to the stdin
of another program. For example, we can combine cat
and grep
to find something in a given file:
# cat reads the package.json file and sends it to stdout
# the pipe commands takes that and sends it as input to grep
# grep searches for any line that has "author" in it and prints it
$ cat package.json | grep "author"
Bang!
The bang !
in linux shells is normally used to refer to previous commands in the command-line history. In zsh you can use it in much the same way with a small difference, !
in zsh are expanded to whatever they refer. This is useful so that you can be more certain of what you’re actually about to execute. For instance, !!
normally refers to the last command in your shell history. In zsh
if you type !!
followed by space
or TAB
it will be expanded to that last command:
# We type this command
$ ls -al
# Then this one
$ !!
# TAB and... It expands to the last command executed
$ ls -al
Here are other useful !
commands:
$ !{char} # expands to last command
$ cat hello.txt
$ !c # expands to the above
$ !! # expands to last command
$ ls -ll
$ !! # expands to the above
$ !$ # expands to the last commands' arguments
Useful unix programs
ls
: list files in the filesystemcat
: print and concatenate fileshead
: print first parts of a filetail
: print last parts of a filegrep
: search text using regexegrep
: search text using extended regular expressions- pagers like
more
,less
andmost
lets you read, navigate and search paginated text interactively. The latter are improved versions. cal
: prints calendar informationsort
: sorts lines of text filesuniq
: report or omit repeated lines
Recipes
grep
# grep -v excludes lines matching a regular expression
$ ls /bin /usr/bin | grep -v '/bin:$' > proglist
# grep -e allows for multiple patterns
# the '^$' regex matches empty lines of text
$ ls /bin /usr/bin | grep -v -e '/bin:$' -e '^$' | sort > proglist
Fuzzy Search in the Command line history and file system
Zsh comes with a really great way to interact with you command line history: forward and backward interactive search. Use C-R
to search backwards in your command line history and C-S
to do the same thing forwards. It has a limitation though, it only shows one single command a time. fzf is a fuzzy finder that integrates with zsh and enhances it in many different ways. One such way is providing amazing support for fuzzy search in you command line history:
Another is providing fuzzy completion for your file system:
To install fzf follow the instructions in the github repository. If you are using MacOS, you can install it using homebrew:
brew install fzf
# To install useful key bindings and fuzzy completion:
$(brew --prefix)/opt/fzf/install
Interesting Zsh Plugins
oh my zsh is a framework for the zsh terminal that helps you manage your zsh configuration through plugins and themes. Oh my zsh comes with hundreds of plugins bundled that you can activate with one line of code in your .zshrc
:
plugins=(
git
zsh-syntax-highlighting
zsh-autosuggestions
vi-mode
)
Here are some of my favorite plugins:
- git: Provides tons of git aliases and a handful of useful functions.
- Syntax highlighting: Provides really nice syntax highlighting right within your zsh terminal.
- Autocompletion suggestions: Provides autosuggestions that you can expand with the right arrow or end. It’s fully customizable.
- Vi mode: Enables vi mode in Zsh, adds a bunch of key bindings and the curent mode to the prompt.
Cool fonts and color schemes for iTerm2
- iTerm 2 color schemes. Download a color scheme and within iTerm2 go to profile, colors, import colors, select the file with the color scheme and it will become available in the collection of themes.
- FiraCode. You’ll need to clone the repo and install the font in TrueType (ttf) format in your mac. Then you’ll be able to enable it within iTerm2.
Great companions for Zsh
References
- Zsh
- Oh my Zsh
- Oh my Zsh themes
- Articles
- Books
- Courses
- Curated GitHub lists
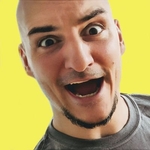
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.