Barbaric Development Toolbox: Elevate Your Front-end Workflow With Yeoman
The Mastering the Arcane Art of JavaScript-mancy series are my humble attempt at bringing my love for JavaScript to all other C# developers that haven’t yet discovered how awesome this language and its whole ecosystem are. These articles are excerpts of the super duper awesome JavaScript-Mancy book a compendium of all things JavaScript for C# developers.
From the experience that I have gathered to this date as a web developer, working and playing with stuff like ASP.NET MVC, RoR, node.js, knockout, angular, etc, I have found that Yeoman - the web scaffolding tool for modern web apps - provides the most awesome front-end web development experience from anything I have ever seen or used by far.
I haven’t worked with it as much as I would’ve liked, nor as deep as I would’ve enjoyed, so I thought it was about time to do something to correct that. You are more than welcome to join me: Let’s improve our front-end development FU and kick some ass with the Yeoman.
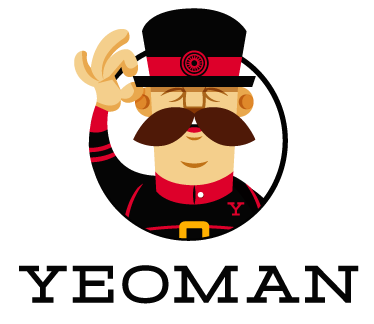
I have divided these series in the following articles. The idea is to start with a brief introduction to the whole Yeoman workflow, I will then continue investigating each particular tool of the stack in more depth, to finally wrap it all up with how you can integrate the workflow with Visual Studio. Hope you like it!!
- Introduction to Yeoman: Yo, Grunt, Gulp, Bower and npm
- Yo, scaffolding the boilerplate for you
- Bower, NuGet for the Front-end
- Grunt, front-end dev ops
- Gulp, like Grunt but different
- Yeoman and Visual Studio
What is Yeoman?
Yeoman is a set of tools that aim to make your life easier as a web developer by automating every possible tedious step and creating a friction-free development environment so that you can focus in what’s important: developing your web app. These steps comprise for instance:
- Creating a whole new project from scratch in seconds
- Live reloading the browser whenever you change your code
- Installing and managing dependencies to third-party libraries and wiring them in your application for you
- Running your tests automagically when you change your code
- Creating components of a web application and wiring them inside your app
- Creating distribution packages with minification, uglifying, image compression, etc
Yeoman itself is compounded by three set of tools:
- yo, the scaffolding tool, helps you create apps from scratch and scaffold - generate code for - other web application components, such as directives in angular or components in knockout.
- bower and npm, the package managers help you install and maintain the libraries you cannot live without. Bower takes care of your front-end libraries while npm focuses on backend and dev tools.
- grunt or gulp the task running tools manage the automation of daily tasks such as building, running tests, hosting a web server so you can preview your web app, etc.
Ok, and now let’s see this in action with a preview of what we will be learning in these articles:
Setting Up Yeoman
In order to setup Yeoman you will need to start by installing node.js. node which started as a platform for developing all sorts of applications in JavaScript, has spawned an amazing community and ecosystem around itself that has fostered collaboration between JavaScript developers and which has, in turn, improved the web development world as a whole. And, as a sort of side effect, node has become an incredible environment for front-end dev-ops tools like yeoman.
Windows
Beware!! The yeoman workflow makes heavy use of a command line. I recommend you to take a look at cmder and PowerShell.
- Get chocolatey, the Windows package manager
- Open your PowerShell console and install node with chocolatey
# cinst is a shortcut for chocolatey install
PS> cinst nodejs.install
- Install yeoman: yo, bower, grunt
# install yo bower and the grunt command line interface globally (-g)
PS> npm install -g yo bower grunt-cli
Mac
If you are a Mac user you are probably familiar with the terminal. If you haven’t heard about tmux, take a look at it, it is a pretty good way to manage several terminals at once.
- Get homebrew, the Mac package manager
- On your terminal (bash, zsh, etc)
$ brew install node
- Install yeoman:
# install yo bower and the grunt command line interface globally (-g)
$ npm install -g yo bower grunt-cli
Creating Some Apps
Ok, so now that we have installed yeoman, let’s create some web apps! During these series I will create a couple of applications with angular.js and knockout.js and you and I will go learning more interesting stuff about yeoman as we go developing them further on each article.
Let’s start with angular.
Creating an Angular App
To create a new angular application with yeoman start by getting the angular generator - a generator is something like a recipe that tells yo how to create a new project (note that there are a lot of generators available for creating different types of applications).
PS> npm install -g generator-angular
Now you can use yo to create a new angular project (say Yes to everything during the installation):
PS> mkdir myFavoriteQuotes
PS> cd myFavoriteQuotes
PS> yo angular
This will create a angular project with the following structure:
app # your app's actual source code
-- scripts # javascript files
-- styles # styles
-- views # partial views
-- images # images
-- favicon.ico # website icon
-- index.html # main page
-- 404.html # 404 not found page
-- robots.txt # robots.txt file for crawlers
test # scaffolded tests for your app
-- spec # a folder for all your jasmine specs
-- karma.conf.js # the karma test runner configuration file
bower_components # here reside all libraries you install via bower
node_modules # here reside all libraries you install via npm
package.json # the definition of our application and its dependencies for node.js and npm
bower.json # a description of our library dependencies in bower
Gruntfile.js # a collection of automated grunt tasks for our enjoyment
.bowerrc # bower configuration
.gitattributes # git repository configuration
.gitignore # files ignored by git
.editorconfig # shared editor configuraiton
.jshintrc # jshint configuration
.travis.yml # can be used with travis CI
You can now preview your application in the browser by using the following command which will essentially start a node.js server and open your browser:
PS> grunt serve
And that’s it for now, you got yourself a basic angular app to start working on. If you want to stop the server you can just click CTRL
+C
on the command line window.
If you can’t wait and want to know more right now, you can find out about the features of the angular generator at GitHub and follow this great tutorial.
Creating a Knockout.js App
Steven Sanderson wrote this yeoman knockout.js generator that lets you start writing a new single page application with a pretty sweet knockout.js setup. Just install the generator by typing:
# Install the knockout generator globally
# and a simple http-server to serve the web app
# since this generator doesn't come with a serve task
PS> npm install -g generator-ko http-server
And then create your new app:
PS> mkdir myFavoriteRecipes
PS> cd myFavoriteRecipes
PS> yo ko
You can either choose to work with TypeScript or JavaScript, and whether or not to add a test setup. When you complete the scaffolding of your new app you will have a folder structure that will like this:
src # a folder containing the source code of your app
-- bower_modules # third party libraries
-- components # knockout components
-- app # your app startup, routing and module configuration
-- css # styles
-- index.html # your app's main page
test # js test folder
node_modules # folder with npm packages
bower.json # bower file with project dependencies
.bowerrc # bower configuration
package.json # npm package information
gulpfile.js # gulp file with all your configured gulp tasks
karma.conf.js # karma test runner configuration
.gitignore # files ignored by git
The generator is configured to help you create a knockout SPA based on knockout components using require.js as module library. You can preview the app in your browser by using the http-server
tool and pointing it to the src
folder:
PS> http-server src
Starting up http-server, serving src on: http://0.0.0.0:8080
Hit CTRL-C to stop the server
This time you’ll need to open a browser yourself (by default localhost:8080
).
Summary
In this article I have introduced yeoman, the superwonderfullytastic web’s scaffolding tool for modern web apps. I hoped you liked it, or found it a little bit interesting and intriguing. Stay tuned if you want to learn some more…
… And if you cannot wait, don’t let me stop you, go on and check these articles:
- Awesome yeoman tutorial
- Getting Started with yeoman
- Getting Started with Bower
- Getting Started with Grunt
- Getting Started with Gulp
- Task Runner Explorer For Visual Studio
Interested in Learning More JavaScript? Buy the Book!
Are you a C# Developer interested in learning JavaScript? Then take a look at the JavaScript-mancy book, a complete compendium of JavaScript for C# developers.
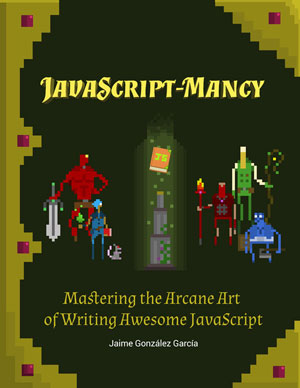
Have a great week ahead!! :)
More Articles In This Series
- Chapter 0: The Basic Ingredients of JavaScriptMancy
- Chapter 1: The Many a One JavaScript Quirks
- Functions
- Object Oriented Programming
- Basics
- ES6
- OOP
- Introduction to OOP in JavaScript for C# Developers
- Summoning Fundamentals: Introduction to OOP In JavaScript – Encapsulation
- Summoning Fundamentals: Introduction to OOP In JavaScript – Inheritance
- Summoning Fundamentals: Introduction to OOP In JavaScript – Polymorphism
- White Tower Summoning: Mimicking C# Classical Inheritance in Javascript
- White Tower Summoning Enhanced: The Marvels of ES6 Classes
- Black Tower Summoning: Object Composition with Mixins
- Safer JavaScript Composition With Traits and Traits.js
- JavaScript Ultra Flexible Object Oriented Programming with Stamps
- Object Oriented JavaScript for C# Programmers
- Tooling
- Data Structures
- Functional Programming
- And more stuff to be shaped out:
- Functional Programming with JavaScript
- Modules
- Asynchronous Programming
- The JavaScript Ecosystem
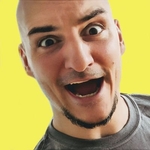
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.