Dev Talk Monday: Douglas Crockford, ES6, New Ways Of Writing Better JavaScript and The Future
Dev Talk Monday is the series that brings you awesome dev talks every Monday
Gooood Monday Vietnaaaam!! :)
Today I bring you a super duper awesome talk from the mighty Douglas Crockford during nordicjs in which he talks about his favorite (and least favorite) features of the upcoming ES 6 Harmony, and how the programming languages of the future may look like.
There is a ton of interesting things in this talk and I wholeheartedly recommend you to watch it not only in order to learn new stuff but for the sheer entertainment of it. Douglas is hilarious as usual. These were my favorite things from the talk:
Much like the params keyword in C#, ES6 will provide the new ellipsis (rest, spread) notation which will bring a real Array
typed variable to handle a varying number of arguments (goodbye old arguments variable):
// Example 1.
// Add function factory.
function add(...args) {
return function(...moreArgs) {
return (
args.reduce(function(a, b) {
return a + b
}) +
moreArgs.reduce(function(a, b) {
return a + b
})
)
}
}
var add2 = add(2)
var add4 = add(2, 2)
console.log(add2(2)) // 4
console.log(add2(4)) // 6
console.log(add4(4)) // 8
// Example 2.
// curry function (partial application)
function curry(fn, ...first) {
return function(...second) {
return fn(...first, ...second)
}
}
function add3Numbers(a, b, c) {
return a + b + c
}
var add5ToTwoNumbers = curry(add3Numbers, 5)
var add10 = curry(add5ToTwoNumbers, 5)
var add100 = curry(add3Numbers, 50, 50)
console.log(add3Numbers(1, 2, 3)) // 6
console.log(add5ToTwoNumbers(5, 5)) // 15
console.log(add10(10)) // 20
console.log(add100(100)) // 200
ES 6 finally brings modules to JavaScript:
export const sqrt = Math.sqrt;
export function square(x) {
return x * x;
}
export function cube(x) {
return square(x)*x;
}
import { square, cube } from 'lib';
console.log(square(2)); // 4
console.log(cube(2)); // 8
import * from as lib from 'lib'
console.log(square(3)); // 9
console.log(cube(3)); // 27
The let keyword which provides block scope to variables (as opposed to function scope), and which will allow destructuring when initializing variables:
let { age, height } = person
// equivalent to
let age = person.age,
height = person.height
Then Douglas starts discussing about the new class keyword and syntax, about object oriented programming, prototypical inheritance and finally comes to a very interesting way of doing OOP through object composition and class-free object oriented programming.
But before I get into that I wanted to make a small remark: it is beyond awesome how he is always questioning the way he programs and tries to improve in any way possible, even forsaking some of the features of a language because they bring more problems than advantages. Things like the new keyword, or the use of this which I find particularly funny because I remember myself reading Secrets of the JavaScript Ninja not long ago, seeing the different behaviors of this, and just thinking: “Boy, there are ways to shoot yourself in the foot with JavaScript. Well, well… I just need to learn and remember it and all will be fine…”. But not for an instant did I stop to think, “perhaps I shouldn’t use _this, perhaps there’s a better way to work with objects in JavaScript”_.
Back to object composition and class-free inheritance. The reasoning goes like this: In class based programming languages you need to create a taxonomy for the different types of objects in your domain, and normally you do this when you know the least about your domain. This means that you are going to do your taxonomy wrong, the wrongness is going to propagate from base to derived classes, you are going to need to fix it, refactor your code and it’s going to be costly. As it turns out, if you throw away classes these problems go away. Then he talks about prototypical inheritance, where objects inherit from objects - prototypes - instead of classes. The main reason to use prototypical inheritance being memory conservation, and this no longer being an issue today, we get to class-free OOP. Thought provoking… XD.
Check this out:
// spec is an object I use as specification
// for my new object type
// It is just a dto for the constructor arguments
// By being an object you can specify arguments in whichever order you like
// add defaults as you please, etc, it is very flexible
function constructor(spec){
// 1. initialize own members from spec
let {member} = spec;
// 2. composition with other objects
// you can select only the parts that you want to use
// you only "inherit" the stuff that you need
let {other} = other_constructor(spec);
// 3. methods
let method = function(){ // close over other methods, variables and spec };
// 4. Expose public API
// Note new ES6 that lets you define object properties like this:
// {method, other}
// instea of
// { method : method, other : other}
return Object.freeze({ // immutable (see https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Global_Objects/Object/freeze)
method,
other
});
}
I would swear that this still produces a taxonomy, albeit much more flexible, but perhaps I haven’t arrived to the uber enlightment level yet. It does fix the problems with this an new though.
And I think that’s enough, go watch the talk itself! :)
P.S. Btw, if you want to start writing ES6 today take a look at this awesome project at GitHub Traceur and, if you just want to tinker check this Chrome extension ES6 REPL.
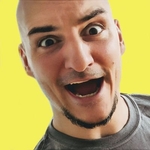
Written by Jaime González García , dad, husband, software engineer, ux designer, amateur pixel artist, tinkerer and master of the arcane arts. You can also find him on Twitter jabbering about random stuff.